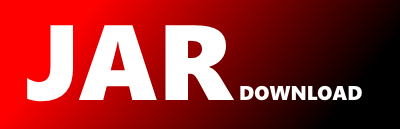
net.chestmc.common.extensions.Server.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package net.chestmc.common.extensions
import org.bukkit.*
import org.bukkit.command.ConsoleCommandSender
import org.bukkit.entity.Player
import org.bukkit.plugin.PluginManager
import org.bukkit.plugin.ServicesManager
import org.bukkit.plugin.messaging.Messenger
import org.bukkit.scheduler.BukkitScheduler
import java.util.*
/**
* Gets the current server of the plugin.
*/
inline val server: Server get() = Bukkit.getServer()
/**
* Gets all online players of this server.
*/
inline val players: Collection get() = Bukkit.getOnlinePlayers()
/**
* Gets all offline players of this server.
*/
inline val offlinePlayers: Array get() = Bukkit.getOfflinePlayers()
/**
* Gets all banned players of this server.
*/
inline val bannedPlayers: Set get() = Bukkit.getBannedPlayers()
/**
* Gets all worlds loaded of this server.
*/
inline val worlds: List get() = Bukkit.getWorlds()
/**
* Gets the [ConsoleCommandSender] of this server.
*/
inline val console: ConsoleCommandSender get() = Bukkit.getConsoleSender()
/**
* Gets the IP of this server.
*/
inline val ip: String get() = Bukkit.getIp()
/**
* Gets the current version of this server.
*/
inline val version: String get() = Bukkit.getVersion()
/**
* Gets the current bukkit version of this server.
*/
inline val bukkitVersion: String get() = Bukkit.getBukkitVersion()
/**
* Gets the whitelist of this server.
*/
inline var whitelist: Boolean
get() = Bukkit.hasWhitelist()
set(value) = Bukkit.setWhitelist(value)
/**
* Gets the MOTD of this server.
*/
inline val motd: String get() = Bukkit.getMotd()
/**
* Gets the plugin manager of this server.
*/
inline val pluginManager: PluginManager get() = Bukkit.getPluginManager()
/**
* Gets the messenger of this server.
*/
inline val messenger: Messenger get() = Bukkit.getMessenger()
/**
* Gets the services manager of this server.
*/
inline val servicesManager: ServicesManager get() = Bukkit.getServicesManager()
/**
* Gets the server name.
*/
inline val serverName: String get() = Bukkit.getServerName()
/**
* Gets the scheduler of this server.
*/
inline val scheduler: BukkitScheduler get() = Bukkit.getScheduler()
/**
* Dispatchs the specified command in the console.
*/
fun dispatchCommand(command: String) = Bukkit.dispatchCommand(console, command)
/**
* Dispatchs all specifieds commands in the console.
*/
fun dispatchCommands(commands: Collection) = commands.onEach {
dispatchCommand(it)
}
/**
* Logs a message to console of this server.
*/
fun log(message: Any) = console.log("§f$message")
/**
* Logs a error message to console of this server.
*/
fun logError(message: Any) = console.log("§cERROR §7- §f$message")
/**
* Logs a warning message to console of this server.
*/
fun logWarning(message: Any) = console.log("§eWARNING §7- §f$message")
/**
* Logs a broadcast message to all players of this server.
*/
fun broadcast(message: Any) = Bukkit.broadcastMessage("$message")
/**
* Gets a player by UUID in this server.
*/
fun player(id: UUID): Player = Bukkit.getPlayer(id)
/**
* Gets a player by name in this server.
*/
fun player(name: String): Player = Bukkit.getPlayer(name)
/**
* Gets a offline player by UUID in this server.
*/
fun offlinePlayer(id: UUID): OfflinePlayer = Bukkit.getOfflinePlayer(id)
/**
* Gets a offline player by name in this server.
*/
fun offlinePlayer(name: String): OfflinePlayer = Bukkit.getOfflinePlayer(name)
/**
* Gets a world by UUID in this server.
*/
fun world(id: UUID): World = Bukkit.getWorld(id)
/**
* Gets a world by name in this server.
*/
fun world(name: String): World = Bukkit.getWorld(name)
/**
* Creates a new location with the specified world, x, y and z.
*/
fun localization(world: World, x: Number, y: Number, z: Number): Location =
Location(world, x.toDouble(), y.toDouble(), z.toDouble())
/**
* Creates a new location with the specified world name, x, y and z.
*/
fun localization(world: String, x: Number, y: Number, z: Number): Location =
Location(world(world), x.toDouble(), y.toDouble(), z.toDouble())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy