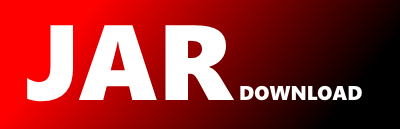
net.chestmc.common.extensions.numbers.Formatters.kt Maven / Gradle / Ivy
package net.chestmc.common.extensions.numbers
import net.chestmc.common.extensions.transform
import java.text.DecimalFormat
import java.util.*
import java.util.concurrent.TimeUnit
/**
* Returns a [DecimalFormat] with a maximum fraction digits of 2.
*/
val DecimalFormatter by lazy {
DecimalFormat("##,###").transform { maximumFractionDigits = 2 }
}
/**
* Returns a [TreeMap] thats corresponds all suffixes to format.
*/
val FormatterSuffixes by lazy {
sortedMapOf(1E3 to "K", 1E6 to "M", 1E9 to "B", 1e12 to "T", 1e15 to "Q", 1e18 to "QQ", 1e21 to "S",
1e24 to "SS", 1E27 to "O", 1e30 to "N", 1e33 to "D", 1e36 to "UN", 1e39 to "DD", 1e42 to "TRD",
1e45 to "QD", 1e48 to "QND", 1e51 to "SD", 1e54 to "SPD", 1e57 to "OCD", 1e60 to "UND", 1e63 to "VG"
) as TreeMap
}
/**
* Returns this number formated as spaced, like:
* `1.000`, `15.591`
*/
fun Number.spaced(): String = DecimalFormatter.format(toDouble())
/**
* Returns this string number as unspaced.
*/
fun String.unspaced() = replace(".", "")
/**
* Returns this number formated with suffixes, like:
* `1K`, `15.59K`
*/
fun Number.format(): String {
val value = toDouble()
if (value < 1000) return "$value"
val entry = FormatterSuffixes.floorEntry(value)
val number = value / (entry.key / 10) / 10
return number.spaced() + entry.value
}
/**
* Returns this number formated as time. The number is parsed as milliseconds.
*/
fun Number.formatTime(): String {
val time = toLong()
if (time <= 0)
return "0s"
val days = TimeUnit.MILLISECONDS.toDays(time)
val hours = TimeUnit.MILLISECONDS.toHours(time) - days * 24
val minutes = TimeUnit.MILLISECONDS.toMinutes(time) - TimeUnit.MILLISECONDS.toHours(time) * 60
val seconds = TimeUnit.MILLISECONDS.toSeconds(time) - TimeUnit.MILLISECONDS.toMinutes(time) * 60
val sb = buildString {
if (days > 0) append(days).append('d').append(' ')
if (hours > 0) append(hours).append('h').append(' ')
if (minutes > 0) append(minutes).append('m').append(' ')
if (seconds > 0) append(seconds).append('s')
}
return sb.ifEmpty { "0s" }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy