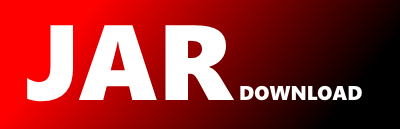
net.chestmc.common.extensions.numbers.Maths.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package net.chestmc.common.extensions.numbers
import java.math.RoundingMode
import kotlin.math.max
import kotlin.math.min
/**
* Returns a random number, specified by the [Math.random]
*/
fun random(): Double = Math.random()
/**
* Returns a random number between two adjacents min and max.
*/
fun randomBetween(min: Int, max: Int): Int = (min + (max - min) * random()).toInt()
/**
* Returns a random number between two adjacents min and max.
*/
fun randomBetween(min: Float, max: Float): Float = (min + (max - min) * random()).toFloat()
/**
* Returns a random number between two adjacents min and max.
*/
fun randomBetween(min: Double, max: Double): Double = min + (max - min) * random()
/**
* Returns the percentage needed to the [min] needs to get in [max].
*/
fun percentage(min: Number, max: Number) = min.toDouble() * 100 / max.toDouble()
/**
* Returns a number between two adjacents min and max.
* This is equals to
* ```
* max(min, min(number, max))
* ```
*/
fun between(min: Int, number: Int, max: Int): Int = max(min, min(number, max))
/**
* Returns a number between two adjacents min and max.
* This is equals to
* ```
* max(min, min(number, max))
* ```
*/
fun between(min: Float, number: Float, max: Float): Float = max(min, min(number, max))
/**
* Returns a number between two adjacents min and max.
* This is equals to
* ```
* max(min, min(number, max))
* ```
*/
fun between(min: Double, number: Double, max: Double): Double = max(min, min(number, max))
/**
* Plus a percent of this actual number.
*/
fun Number.plusPercent(percent: Double): Double = toDouble() + toDouble() / 100 * percent
/**
* Subtract a percent of this actual number.
*/
fun Number.minusPercent(percent: Double): Double = toDouble() - toDouble() / 100 * percent
/**
* Multiplies a percent of this actual number.
*/
fun Number.timesPercent(percent: Double): Double = toDouble() * toDouble() / 100 * percent
/**
* Divides a percent of this actual number.
*/
fun Number.divPercent(percent: Double): Double = toDouble() / toDouble() / 100 * percent
/**
* Rounds this number to a max decimal places.
*/
fun Number.round(decimals: Int): Double =
toDouble().toBigDecimal().setScale(decimals, RoundingMode.HALF_UP).toDouble()
/**
* Returns true if a random number multiplied by 100 is below or equals
* than [of], or false if otherwise.
*/
fun chance(of: Double): Boolean = random() * 100 <= of
© 2015 - 2025 Weber Informatics LLC | Privacy Policy