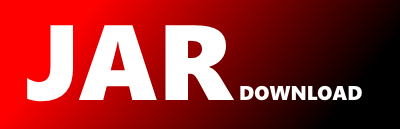
net.chestmc.common.interfaces.Localizable.kt Maven / Gradle / Ivy
package net.chestmc.common.interfaces
import io.github.uinnn.interfaces.common.Filter
import net.chestmc.common.MinecraftEntity
import net.chestmc.common.extensions.getRelative
import net.chestmc.common.extensions.handler
import net.chestmc.common.extensions.nearbyEntities
import org.bukkit.Location
import org.bukkit.Material
import org.bukkit.block.Block
import org.bukkit.entity.Entity
import org.bukkit.entity.Player
import java.util.*
/**
* A localizable interface, representing a object that have a location.
*/
interface Localizable {
/**
* The location of this localizable.
*/
var location: Location
}
/**
* Returns if the location of this localizable object is nearby to another
* location in the specified radius.
*/
fun Localizable.isNear(to: Location, radius: Int): Boolean {
for (x in -radius until radius) {
for (y in -radius until radius) {
for (z in -radius until radius) {
val relative = location.getRelative(x, y, z)
if (to.blockX == relative.blockX && to.blockY == relative.blockY && to.blockZ == relative.blockZ)
return true
}
}
}
return false
}
/**
* Returns if the location of this localizable object is nearby to location of
* the specified entity in the specified radius.
*/
fun Localizable.isNear(to: Entity, radius: Int): Boolean = isNear(to.location, radius)
/**
* Returns if the location of this localizable object is nearby to location of
* the specified block in the specified radius.
*/
fun Localizable.isNear(to: Block, radius: Int): Boolean = isNear(to.location, radius)
/**
* Returns the nearest entities in the specified radius as list
*/
fun Localizable.nearbyEntities(radius: Int): List =
location.nearbyEntities(radius, radius, radius).toList()
/**
* Returns the nearest entity in the specified radius
*/
fun Localizable.nearestEntity(radius: Int): Entity? = nearbyEntities(radius).firstOrNull()
/**
* Returns the nearest entities filtered in the specified radius as list
*/
inline fun Localizable.nearbyEntities(radius: Int, predicate: Filter): List =
location.nearbyEntities(radius, radius, radius).filter(predicate).toList()
/**
* Returns the nearest entity filtered in the specified radius
*/
inline fun Localizable.nearestEntity(radius: Int, predicate: Filter): Entity? =
nearbyEntities(radius, predicate).firstOrNull()
/**
* Returns the nearest entities filtered in the specified radius as list
*/
@JvmName("nearbyEntitiesInstance")
inline fun Localizable.nearbyEntitiesHandlers(radius: Int): List =
nearbyEntities(radius).map(Entity::handler).filterIsInstance()
/**
* Returns the nearest entity filtered in the specified radius
*/
@JvmName("nearestEntityInstance")
inline fun Localizable.nearestEntityHandler(radius: Int): T? =
nearbyEntitiesHandlers(radius).firstOrNull()
/**
* Returns the nearest entities filtered in the specified radius as list
*/
@JvmName("nearbyEntitiesType")
inline fun Localizable.nearbyEntities(radius: Int): List =
nearbyEntities(radius).filterIsInstance()
/**
* Returns the nearest entity filtered in the specified radius
*/
@JvmName("nearestEntityType")
inline fun Localizable.nearestEntity(radius: Int): T? =
nearbyEntities(radius).firstOrNull()
/**
* Returns the nearest players filtered in the specified radius as list
*/
fun Localizable.nearbyPlayers(radius: Int): List =
nearbyEntities(radius).filterIsInstance()
/**
* Returns the nearest player filtered in the specified radius
*/
fun Localizable.nearestPlayer(radius: Int): Player? = nearbyPlayers(radius).firstOrNull()
/**
* Returns all nearby blocks centered by the location of this localizable object.
*/
fun Localizable.nearbyBlocks(radius: Int): List = LinkedList().apply {
for (x in -radius until radius) {
for (y in -radius until radius) {
for (z in -radius until radius) {
val relative = location.getRelative(x, y, z)
if (location == relative) continue
add(relative.block)
}
}
}
}
/**
* Returns all filtered nearby blocks centered by the location of this localizable object.
*/
inline fun Localizable.nearbyBlocks(radius: Int, predicate: Filter): List =
nearbyBlocks(radius).filter(predicate)
/**
* Returns all filtered nearby blocks centered by the location of this localizable object.
*/
fun Localizable.nearbyBlocks(radius: Int, type: Material): List = nearbyBlocks(radius) {
it.type == type
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy