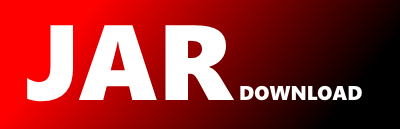
net.chestmc.common.metadata.Metadatable.kt Maven / Gradle / Ivy
package net.chestmc.common.metadata
import net.jodah.expiringmap.ExpirationPolicy
import net.jodah.expiringmap.ExpiringMap
import java.util.concurrent.TimeUnit
typealias Metadata = ExpiringMap
/**
* A metadatable interface represents thats the object
* may contains any metadata, this is, storing any type of object with a key.
*/
interface Metadatable {
/**
* All registered metadata of this metadatable object.
*/
var metadata: Metadata
/**
* Interject a metadata, this is,
* put a data or overwrite a existent data.
*/
fun interject(key: String, value: T): T {
metadata.put(key, value, ExpirationPolicy.CREATED)
return value
}
/**
* Interject a metadata, this is,
* put a data or overwrite a existent data.
*/
fun interject(
key: String,
value: T,
duration: Long,
unit: TimeUnit,
policy: ExpirationPolicy = ExpirationPolicy.CREATED
): T {
metadata.put(key, value, policy, duration, unit)
return value
}
/**
* Introduce a metadata, this is,
* put a data only if not exists in the storage.
*/
fun introduce(key: String, value: T): T {
metadata.putIfAbsent(key, value)
return value
}
/**
* Returns if the specified key is in the storage
* of this metadata object.
*/
fun includes(key: String) = metadata.containsKey(key)
/**
* Invalidates a metadata, this is,
* remove a existent metadata in the storage.
*/
fun invalidate(key: String) {
metadata.remove(key)
}
/**
* Resets the expiration time of a key.
*/
fun resetExpiration(key: String) = metadata.resetExpiration(key)
/**
* Gets the expirition time of this key.
*/
fun expiresIn(key: String): Long = metadata.getExpiration(key)
/**
* Gets the expected expirition time of this key.
*/
fun expectedExpiresIn(key: String): Long = metadata.getExpectedExpiration(key)
/**
* Try locates a existent metadata, or
* null if the metadata not exists in the storage.
*/
fun locate(key: String): T? = metadata[key]
/**
* Acquire a existent metadata as a [Result] of [T].
* Supporting this was safe try-catch use.
*/
fun acquire(key: String): Result = runCatching {
metadata[key]!!
}
/**
* Adds a listener to be triggered when a key expires.
* This runs synchronously.
*/
fun addExpirationListener(action: (String, T) -> Unit) {
metadata.addExpirationListener(action)
}
/**
* Adds a listener to be triggered when a key expires.
* This runs asynchronously.
*/
fun addAsyncExpirationListener(action: (String, T) -> Unit) {
metadata.addAsyncExpirationListener(action)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy