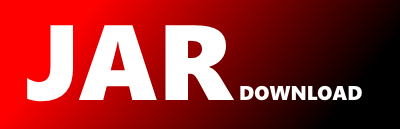
net.chestmc.common.registry.Registry.kt Maven / Gradle / Ivy
package net.chestmc.common.registry
/**
* A registry represents a key-value holder object.
* This can be used to stores data.
*/
interface Registry : MutableMap {
/**
* The holder mutable map of this registry.
*/
val delegate: MutableMap
/**
* Register a key-value to this registry.
*/
fun submit(key: K, value: V): V? = delegate.put(key, value)
/**
* Unregister a key of this registry.
*/
fun unsubmit(key: K): V? = delegate.remove(key)
/**
* Try locates a existent value, or
* null if the value not exists in the storage.
*/
fun locate(key: K): V? = delegate[key]
/**
* Acquire a existent value as a [Result].
* Supporting this was safe try-catch use.
*/
fun acquire(key: K): Result = runCatching {
delegate[key]!!
}
/*
* delegation
*/
override val size: Int get() = delegate.size
override val entries: MutableSet> get() = delegate.entries
override val keys: MutableSet get() = delegate.keys
override val values: MutableCollection get() = delegate.values
override fun put(key: K, value: V): V? = submit(key, value)
override fun remove(key: K): V? = unsubmit(key)
override fun containsKey(key: K): Boolean = delegate.containsKey(key)
override fun containsValue(value: V): Boolean = delegate.containsValue(value)
override fun get(key: K): V = delegate[key]!!
override fun isEmpty(): Boolean = delegate.isEmpty()
override fun clear() = delegate.clear()
override fun putAll(from: Map) = from.forEach { (key, value) ->
submit(key, value)
}
}
/**
* Gets a value of this registry by index or throws a exception if the value of
* the specified index not exists.
*/
fun Registry<*, V>.acquire(index: Int): V = values.toList()[index]
/**
* Gets a value of this registry by index or null if the value of
* the specified index not exists.
*/
fun Registry<*, V>.locate(index: Int): V? = values.toList().getOrNull(index)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy