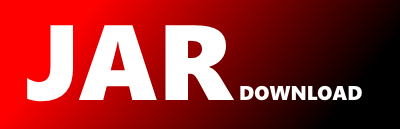
net.chestmc.entity.IEntity.kt Maven / Gradle / Ivy
package net.chestmc.entity
import net.chestmc.common.DamageSource
import net.minecraft.server.NBTTagCompound
import net.minecraft.server.PathfinderGoal
import org.bukkit.Location
import org.bukkit.block.Block
import org.bukkit.entity.Entity
import org.bukkit.entity.Player
/**
* A handler for custom entities. This is a general interface for any entity.
*/
interface IEntity {
/**
* Gets the current location of this entity.
*/
val location: Location
/**
* Gets the bukkit entity related to this entity.
*/
val entity: Entity
/**
* When this entity spawns, this will be called.
* If this function returns false, the entity will not spawn.
*/
fun onSpawn(): Boolean
/**
* When this entity post spawn, this will be called.
*/
fun onPostSpawn()
/**
* When this entity dies, this will be called.
* If this function returns false, the entity will not die.
*/
fun onDie(): Boolean
/**
* When this entity dies by a another entity, this will be called.
* If this function returns false, the entity will not die.
*/
fun onDie(entity: Entity): Boolean
/**
* When a player interacts with this entity, this will be called.
* If this function returns false, the interact action will not be performed.
*/
fun onInteract(player: Player): Boolean
/**
* When a player pre-interacts with this entity, this will be called.
*/
fun onPreInteract(player: Player)
/**
* When a player attacks this entity, this will be called.
* If this function returns false, the attack will not be performed.
*/
fun onAttack(player: Player, damage: Double): Boolean
/**
* When this entity takes any damage, this will be called.
* If this function returns false, the damage will not be performed.
*/
fun onDamage(source: DamageSource, damage: Double): Boolean
/**
* When this entity takes any damage from another entity, this will be called.
* If this function returns false, the damage will not be performed.
*/
fun onDamage(entity: Entity, source: DamageSource, damage: Double): Boolean
/**
* When this entity takes any damage from another block, this will be called.
* If this function returns false, the damage will not be performed.
*/
fun onDamage(block: Block, source: DamageSource, damage: Double): Boolean
/**
* When this entity jumps, this will be called.
* If this functions returns false, the entity will not jump.
*/
fun onJump(block: Block): Boolean
/**
* When this entity post jumps, this will be called.
* This is called after the entity jump, with this you can configure how high the entity will jump.
*/
fun onPostJump(block: Block)
/**
* When this entity falls on a block, this will be called.
*/
fun onFall(block: Block, height: Float)
/**
* When this entity walks on a block, this will be called.
*/
fun onMove(block: Block)
/**
* When this entity ticks, this will be called.
*/
fun onTick()
/**
* When this entity collides with a another entity, this will be called.
*/
fun onCollide(entity: Entity)
/**
* Adds a new goal behavior for this entity.
*/
fun addBehavior(priority: Int, behavior: PathfinderGoal)
/**
* Adds a new target behavior for this entity.
*/
fun addTargetBehavior(priority: Int, behavior: PathfinderGoal)
/**
* Clears all goal behavior of this entity.
*/
fun clearBehaviors()
/**
* Clears all target behavior of this entity.
*/
fun clearTargetBehaviors()
/**
* Saves the data of this entity.
*/
fun save(tag: NBTTagCompound)
/**
* Loads the data of entity.
*/
fun load(tag: NBTTagCompound)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy