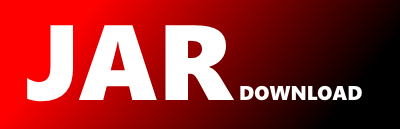
net.chestmc.library.LibraryRegistry.kt Maven / Gradle / Ivy
package net.chestmc.library
import net.chestmc.Downloader
import net.chestmc.common.extensions.newFolder
import net.chestmc.common.registry.AbstractRegistry
import java.io.File
import java.lang.reflect.Method
import java.net.URL
import java.net.URLClassLoader
/**
* A registry used to store and register libraries.
*/
object LibraryRegistry : AbstractRegistry() {
const val MAVEN_CENTRAL = "https://repo.maven.apache.org/maven2/"
/**
* Gets all default dependencies by notation.
*/
val defaultDependencies by lazy {
listOf(
"org.jetbrains.kotlin:kotlin-reflect:1.5.30",
"org.jetbrains.kotlinx:kotlinx-coroutines-core:1.5.1",
"org.jetbrains.kotlinx:kotlinx-serialization-core:1.2.2",
"org.jetbrains.kotlinx:kotlinx-serialization-json:1.2.2",
"org.jetbrains.kotlinx:kotlinx-serialization-protobuf:1.2.2",
"com.charleskorn.kaml:kaml:0.35.2",
"net.benwoodworth.knbt:knbt:0.8.1",
"commons-lang:commons-lang:2.6",
"com.google.guava:guava:17.0",
"joda-time:joda-time:2.10.10",
"net.jodah:expiringmap:0.5.10",
"mysql:mysql-connector-java:5.1.14",
"org.xerial:sqlite-jdbc:3.36.0.1",
"org.avaje:ebean:2.8.1",
"com.zaxxer:HikariCP:4.0.3",
"com.google.code.gson:gson:2.2.4",
"com.googlecode.json-simple:json-simple:1.1.1",
"org.yaml:snakeyaml:1.14",
"net.sf.trove4j:trove4j:3.0.3",
"jline:jline:2.12"
)
}
/**
* Gets the default folder of the libaries.
*/
val folder = newFolder("libraries")
/**
* Loads and register all libraries from the default library folder.
*/
fun loadAll() {
val files = folder.listFiles { file -> file.extension == "jar" }!!
for (file in files) {
if (file.nameWithoutExtension in this)
continue
submit(file.nameWithoutExtension, file)
addURLMethod.invoke(mainClassLoader, file.toURI().toURL())
}
}
/**
* Adds and register a new library by the specified file.
*/
fun register(file: File) {
if (file.nameWithoutExtension in this)
return
file.copyTo(folder)
submit(file.nameWithoutExtension, file)
addURLMethod.invoke(mainClassLoader, file.toURI().toURL())
}
/**
* Downloads a jar file from the specified [url], and implements then inside of the library folder.
* This alsos register the downloaded file in this registry.
*/
fun download(url: String): File {
val file = Downloader.download(URL(url), folder, true)
submit(file.nameWithoutExtension, file)
addURLMethod.invoke(mainClassLoader, file.toURI().toURL())
return file
}
/**
* Downloads a jar file from the maven central and implements then inside of the library folder.
* This alsos register the downloaded file in this registry.
*/
fun downloadFromMaven(group: String, artifact: String, version: String): File {
val url = buildString {
val normalizedArtifact = artifact.replace('.', '/')
append(MAVEN_CENTRAL)
append(group.replace('.', '/')).append('/')
append(normalizedArtifact).append('/')
append(version).append('/')
append(normalizedArtifact).append('-').append(version).append(".jar")
}
return download(url)
}
}
/**
* Gets the main class loader.
*/
internal val mainClassLoader by lazy {
ClassLoader.getSystemClassLoader() as URLClassLoader
}
/**
* Gets the java method used to add new URL libaries to the classpath.
*/
internal val addURLMethod: Method by lazy {
URLClassLoader::class.java.getDeclaredMethod("addURL", URL::class.java).apply {
isAccessible = true
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy