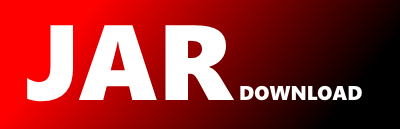
net.chestmc.nbt.InventoryAdapter.kt Maven / Gradle / Ivy
package net.chestmc.nbt
import net.chestmc.Slot
import net.minecraft.server.NBTTagCompound
import net.minecraft.server.NBTTagList
import org.bukkit.Bukkit
import org.bukkit.inventory.Inventory
/**
* A tag adapter for adapting [Inventory].
*/
object InventoryAdapter : TagAdapter {
override fun load(key: String, tag: NBTTagCompound): Inventory {
return Bukkit.createInventory(null, tag.getByte("Size").toInt() * 9, tag.getString("Title")).apply {
extract(this, tag)
}
}
override fun save(key: String, value: Inventory, tag: NBTTagCompound) {
tag.setString("Title", value.title)
tag.setByte("Size", (value.size / 9).toByte())
tag.set("Items", populate(value))
}
fun extract(inventory: Inventory, tag: NBTTagCompound) {
for (compound in tag.getList("Items", NBTTagCompound.COMPOUND)) {
if (compound !is NBTTagCompound) continue
val slot = compound.getSlot("SlotType")
inventory.setItem(slot.slot, slot.item)
}
}
fun populate(inventory: Inventory): NBTTagList = NBTTagList().apply {
for (index in 0 until inventory.size) {
val item = inventory.getItem(index) ?: continue
add(NBTTagCompound().apply {
setSlot("SlotType", Slot(index, item))
})
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy