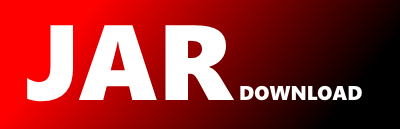
net.chestmc.nbt.ItemAdapter.kt Maven / Gradle / Ivy
package net.chestmc.nbt
import net.chestmc.common.extensions.collections.emptyMutableList
import net.minecraft.server.NBTBase
import net.minecraft.server.NBTTagCompound
import net.minecraft.server.NBTTagList
import org.bukkit.craftbukkit.inventory.CraftItemStack
import org.bukkit.inventory.ItemStack
/**
* A tag adapter for adapting [ItemStack].
*/
object ItemAdapter : TagAdapter {
override fun load(key: String, tag: NBTTagCompound): ItemStack {
return extract(tag.getCompound(key))
}
override fun save(key: String, value: ItemStack, tag: NBTTagCompound) {
populate(key, value, tag)
}
fun populate(key: String, item: ItemStack, tag: NBTTagCompound): NBTTagCompound = tag.apply {
set(key, item.handler.save(NBTTagCompound()))
}
fun extract(tag: NBTTagCompound): ItemStack {
return CraftItemStack.asBukkitCopy(net.minecraft.server.ItemStack.createStack(tag))
}
}
/**
* A tag adapter for adapting a list of [ItemStack].
*/
object ItemListAdapter : TagAdapter> {
override fun load(key: String, tag: NBTTagCompound): MutableList {
return extract(tag.getList(key, NBTBase.COMPOUND), emptyMutableList())
}
override fun save(key: String, value: MutableList, tag: NBTTagCompound) {
tag.set(key, populate(NBTTagList(), value))
}
fun populate(list: NBTTagList, items: List): NBTTagList = list.apply {
for (item in items) {
add(ItemAdapter.populate("ItemStack", item, NBTTagCompound()))
}
}
fun extract(from: NBTTagList, to: MutableList): MutableList = to.apply {
for (compound in from) {
if (compound !is NBTTagCompound)
continue
add(ItemAdapter.extract(compound))
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy