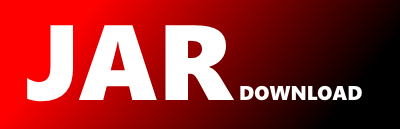
net.minecraft.server.BlockStateList Maven / Gradle / Ivy
package net.minecraft.server;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Objects;
import com.google.common.collect.*;
import java.util.*;
public class BlockStateList {
private static final Joiner a = Joiner.on(", ");
private static final Function b = new Function() {
public String a(IBlockState iblockstate) {
return iblockstate == null ? "" : iblockstate.a();
}
public Object apply(Object object) {
return this.a((IBlockState) object);
}
};
private final Block c;
private final ImmutableList d;
private final ImmutableList e;
public BlockStateList(Block block, IBlockState... aiblockstate) {
this.c = block;
Arrays.sort(aiblockstate, new Comparator() {
public int a(IBlockState iblockstate, IBlockState iblockstate1) {
return iblockstate.a().compareTo(iblockstate1.a());
}
public int compare(Object object, Object object1) {
return this.a((IBlockState) object, (IBlockState) object1);
}
});
this.d = ImmutableList.copyOf(aiblockstate);
LinkedHashMap linkedhashmap = Maps.newLinkedHashMap();
ArrayList arraylist = Lists.newArrayList();
Iterable iterable = IteratorUtils.a(this.e());
Iterator iterator = iterable.iterator();
while (iterator.hasNext()) {
List list = (List) iterator.next();
Map map = MapGeneratorUtils.b(this.d, list);
BlockData blockstatelist_blockdata = new BlockData(block, ImmutableMap.copyOf(map), null);
linkedhashmap.put(map, blockstatelist_blockdata);
arraylist.add(blockstatelist_blockdata);
}
iterator = arraylist.iterator();
while (iterator.hasNext()) {
BlockData blockstatelist_blockdata1 = (BlockData) iterator.next();
blockstatelist_blockdata1.a(linkedhashmap);
}
this.e = ImmutableList.copyOf(arraylist);
}
public ImmutableList a() {
return this.e;
}
private List> e() {
ArrayList arraylist = Lists.newArrayList();
for (int i = 0; i < this.d.size(); ++i) {
arraylist.add(this.d.get(i).c());
}
return arraylist;
}
public IBlockData getBlockData() {
return this.e.get(0);
}
public Block getBlock() {
return this.c;
}
public Collection d() {
return this.d;
}
public String toString() {
return Objects.toStringHelper(this).add("block", Block.REGISTRY.c(this.c)).add("properties", Iterables.transform(this.d, BlockStateList.b)).toString();
}
static class BlockData extends BlockDataAbstract {
private final Block a;
private final ImmutableMap b;
private ImmutableTable c;
private BlockData(Block block, ImmutableMap immutablemap) {
this.a = block;
this.b = immutablemap;
}
BlockData(Block block, ImmutableMap immutablemap, Object object) {
this(block, immutablemap);
}
public Collection a() {
return Collections.unmodifiableCollection(this.b.keySet());
}
public > T get(IBlockState iblockstate) {
if (!this.b.containsKey(iblockstate)) {
throw new IllegalArgumentException("Cannot get property " + iblockstate + " as it does not exist in " + this.a.P());
} else {
return iblockstate.b().cast(this.b.get(iblockstate));
}
}
public , V extends T> IBlockData set(IBlockState iblockstate, V v0) {
if (!this.b.containsKey(iblockstate)) {
throw new IllegalArgumentException("Cannot set property " + iblockstate + " as it does not exist in " + this.a.P());
} else if (!iblockstate.c().contains(v0)) {
throw new IllegalArgumentException("Cannot set property " + iblockstate + " to " + v0 + " on block " + Block.REGISTRY.c(this.a) + ", it is not an allowed value");
} else {
return this.b.get(iblockstate) == v0 ? this : this.c.get(iblockstate, v0);
}
}
public ImmutableMap b() {
return this.b;
}
public Block getBlock() {
return this.a;
}
public boolean equals(Object object) {
return this == object;
}
public int hashCode() {
return this.b.hashCode();
}
public void a(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy