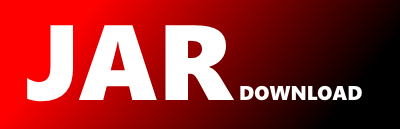
net.minecraft.server.EntityTNTPrimed Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package net.minecraft.server;
import org.bukkit.entity.TNTPrimed;
import org.bukkit.event.entity.ExplosionPrimeEvent;
import org.jetbrains.annotations.NotNull;
public class EntityTNTPrimed extends Entity {
public int fuseTicks;
public float yield = 4; // CraftBukkit - add field
public boolean isIncendiary = false; // CraftBukkit - add field
private EntityLiving source;
public EntityTNTPrimed(World world) {
super(world);
this.k = true;
this.setSize(0.98F, 0.98F);
}
@NotNull
@Override
public TNTPrimed getEntity() {
return (TNTPrimed) super.getEntity();
}
public EntityTNTPrimed(World world, double d0, double d1, double d2, EntityLiving entityliving) {
this(world);
this.setPosition(d0, d1, d2);
float f = (float) (Math.random() * 3.1415927410125732D * 2.0D);
this.motX = -((float) Math.sin(f)) * 0.02F;
this.motY = 0.20000000298023224D;
this.motZ = -((float) Math.cos(f)) * 0.02F;
this.fuseTicks = 80;
this.lastX = d0;
this.lastY = d1;
this.lastZ = d2;
this.source = entityliving;
}
protected void h() {
}
protected boolean s_() {
return false;
}
public boolean ad() {
return !this.dead;
}
public void t_() {
if (world.spigotConfig.currentPrimedTnt++ > world.spigotConfig.maxTntTicksPerTick) {
return;
} // Spigot
this.lastX = this.locX;
this.lastY = this.locY;
this.lastZ = this.locZ;
this.motY -= 0.03999999910593033D;
this.move(this.motX, this.motY, this.motZ);
this.motX *= 0.9800000190734863D;
this.motY *= 0.9800000190734863D;
this.motZ *= 0.9800000190734863D;
if (this.onGround) {
this.motX *= 0.699999988079071D;
this.motZ *= 0.699999988079071D;
this.motY *= -0.5D;
}
if (this.fuseTicks-- <= 0) {
// CraftBukkit start - Need to reverse the order of the explosion and the entity death so we have a location for the event
// this.die();
if (!this.world.isClientSide) {
this.explode();
}
this.die();
// CraftBukkit end
} else {
this.W();
this.world.addParticle(EnumParticle.SMOKE_NORMAL, this.locX, this.locY + 0.5D, this.locZ, 0.0D, 0.0D, 0.0D);
}
}
private void explode() {
// CraftBukkit start
// float f = 4.0F;
org.bukkit.craftbukkit.CraftServer server = this.world.getServer();
ExplosionPrimeEvent event = new ExplosionPrimeEvent((org.bukkit.entity.Explosive) org.bukkit.craftbukkit.entity.CraftEntity.getEntity(server, this));
server.getPluginManager().callEvent(event);
if (!event.isCancelled()) {
this.world.createExplosion(this, this.locX, this.locY + (double) (this.length / 2.0F), this.locZ, event.getRadius(), event.getFire(), true);
}
// CraftBukkit end
}
protected void b(NBTTagCompound nbttagcompound) {
nbttagcompound.setByte("Fuse", (byte) this.fuseTicks);
}
protected void a(NBTTagCompound nbttagcompound) {
this.fuseTicks = nbttagcompound.getByte("Fuse");
}
public EntityLiving getSource() {
return this.source;
}
public float getHeadHeight() {
return 0.0F;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy