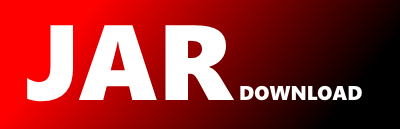
org.bukkit.CropState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package org.bukkit;
import com.google.common.collect.Maps;
import java.util.Map;
/**
* Represents the different growth states of crops
*/
public enum CropState {
/**
* State when first seeded
*/
SEEDED(0x0),
/**
* First growth stage
*/
GERMINATED(0x1),
/**
* Second growth stage
*/
VERY_SMALL(0x2),
/**
* Third growth stage
*/
SMALL(0x3),
/**
* Fourth growth stage
*/
MEDIUM(0x4),
/**
* Fifth growth stage
*/
TALL(0x5),
/**
* Almost ripe stage
*/
VERY_TALL(0x6),
/**
* Ripe stage
*/
RIPE(0x7);
private final static Map BY_DATA = Maps.newHashMap();
static {
for (CropState cropState : values()) {
BY_DATA.put(cropState.getData(), cropState);
}
}
private final byte data;
CropState(final int data) {
this.data = (byte) data;
}
/**
* Gets the CropState with the given data value
*
* @param data Data value to fetch
* @return The {@link CropState} representing the given value, or null if
* it doesn't exist
* @deprecated Magic value
*/
@Deprecated
public static CropState getByData(final byte data) {
return BY_DATA.get(data);
}
/**
* Gets the associated data value representing this growth state
*
* @return A byte containing the data value of this growth state
* @deprecated Magic value
*/
@Deprecated
public byte getData() {
return data;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy