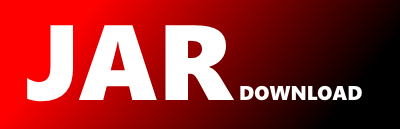
org.bukkit.block.CreatureSpawner Maven / Gradle / Ivy
package org.bukkit.block;
import org.bukkit.entity.CreatureType;
import org.bukkit.entity.EntityType;
/**
* Represents a creature spawner.
*/
public interface CreatureSpawner extends BlockState {
/**
* Gets the min delay to this spawner spawns a entity.
*/
int getMinDelay(); // ChestMC - add method.
/**
* Sets the min delay to this spawner spawns a entity.
*/
void setMinDelay(int delay); // ChestMC - add method.
/**
* Gets the max delay to this spawner spawns a entity.
*/
int getMaxDelay(); // ChestMC - add method.
/**
* Sets the max delay to this spawner spawns a entity.
*/
void setMaxDelay(int delay); // ChestMC - add method.
/**
* Gets the count of entities that this spawn will spawns.
*/
int getCount(); // ChestMC - add method.
/**
* Sets the count of entities that this spawn will spawns.
*/
void setCount(int amount); // ChestMC - add method.
/**
* Gets the max nearby entities amount that this spawner can spawn.
* If the nearby entities around this spawner is higher than the max,
* the spawner will not spawn more entities.
*/
int getMaxNearbyEntities(); // ChestMC - add method.
/**
* Sets the max nearby entities amount that this spawner can spawn.
*/
void setMaxNearbyEntities(int amount); // ChestMC - add method.
/**
* Gets the required player range that this spawner will be able to spawn entities.
*/
int getPlayerRange(); // ChestMC - add method.
/**
* Sets the required player range that this spawner will be able to spawn entities.
*/
void setPlayerRange(int amount); // ChestMC - add method.
/**
* Gets the spawn range that this spawner will spawn entities.
* This is, the higher this value, the farther entities can spawn.
*/
int getSpawnRange(); // ChestMC - add method.
/**
* Sets the spawn range that this spawner will spawn entities.
*/
void setSpawnRange(int amount); // ChestMC - add method.
/**
* Get the spawner's creature type.
*
* @return The creature type.
* @deprecated In favour of {@link #getSpawnedType()}.
*/
@Deprecated
CreatureType getCreatureType();
/**
* Set the spawner creature type.
*
* @param creatureType The creature type.
* @deprecated In favour of {@link #setSpawnedType(EntityType)}.
*/
@Deprecated
void setCreatureType(CreatureType creatureType);
/**
* Get the spawner's creature type.
*
* @return The creature type.
*/
EntityType getSpawnedType();
/**
* Set the spawner's creature type.
*
* @param creatureType The creature type.
*/
void setSpawnedType(EntityType creatureType);
/**
* Get the spawner's creature type.
*
* @return The creature type's name.
* @deprecated Use {@link #getCreatureTypeName()}.
*/
@Deprecated
String getCreatureTypeId();
/**
* Set the spawner mob type.
*
* @param creatureType The creature type's name.
* @deprecated Use {@link #setCreatureTypeByName(String)}.
*/
@Deprecated
void setCreatureTypeId(String creatureType);
/**
* Set the spawner mob type.
*
* @param creatureType The creature type's name.
*/
void setCreatureTypeByName(String creatureType);
/**
* Get the spawner's creature type.
*
* @return The creature type's name.
*/
String getCreatureTypeName();
/**
* Get the spawner's delay.
*
* @return The delay.
*/
int getDelay();
/**
* Set the spawner's delay.
*
* @param delay The delay.
*/
void setDelay(int delay);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy