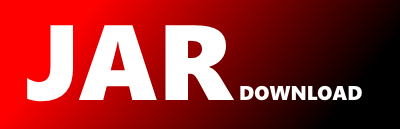
org.bukkit.command.SimpleCommandMap Maven / Gradle / Ivy
package org.bukkit.command;
import org.apache.commons.lang.Validate;
import org.bukkit.Server;
import org.bukkit.command.defaults.*;
import org.bukkit.entity.Player;
import org.bukkit.util.StringUtil;
import java.util.*;
import java.util.regex.Pattern;
import static org.bukkit.util.Java15Compat.Arrays_copyOfRange;
public class SimpleCommandMap implements CommandMap {
private static final Pattern PATTERN_ON_SPACE = Pattern.compile(" ", Pattern.LITERAL);
protected final Map knownCommands = new HashMap();
private final Server server;
public SimpleCommandMap(final Server server) {
this.server = server;
setDefaultCommands();
}
private void setDefaultCommands() {
register("bukkit", new VersionCommand("version"));
register("bukkit", new ReloadCommand("reload"));
register("bukkit", new PluginsCommand("plugins"));
register("bukkit", new TimingsCommand("timings"));
}
public void setFallbackCommands() {
register("bukkit", new HelpCommand());
}
/**
* {@inheritDoc}
*/
public void registerAll(String fallbackPrefix, List commands) {
if (commands != null) {
for (Command c : commands) {
register(fallbackPrefix, c);
}
}
}
/**
* {@inheritDoc}
*/
public boolean register(String fallbackPrefix, Command command) {
return register(command.getName(), fallbackPrefix, command);
}
/**
* {@inheritDoc}
*/
public boolean register(String label, String fallbackPrefix, Command command) {
label = label.toLowerCase().trim();
fallbackPrefix = fallbackPrefix.toLowerCase().trim();
boolean registered = register(label, command, false, fallbackPrefix);
Iterator iterator = command.getAliases().iterator();
while (iterator.hasNext()) {
if (!register(iterator.next(), command, true, fallbackPrefix)) {
iterator.remove();
}
}
// If we failed to register under the real name, we need to set the command label to the direct address
if (!registered) {
command.setLabel(fallbackPrefix + ":" + label);
}
// Register to us so further updates of the commands label and aliases are postponed until its reregistered
command.register(this);
return registered;
}
/**
* Registers a command with the given name is possible. Also uses
* fallbackPrefix to create a unique name.
*
* @param label the name of the command, without the '/'-prefix.
* @param command the command to register
* @param isAlias whether the command is an alias
* @param fallbackPrefix a prefix which is prepended to the command for a
* unique address
* @return true if command was registered, false otherwise.
*/
private synchronized boolean register(String label, Command command, boolean isAlias, String fallbackPrefix) {
knownCommands.put(fallbackPrefix + ":" + label, command);
if ((command instanceof VanillaCommand || isAlias) && knownCommands.containsKey(label)) {
// Request is for an alias/fallback command and it conflicts with
// a existing command or previous alias ignore it
// Note: This will mean it gets removed from the commands list of active aliases
return false;
}
boolean registered = true;
// If the command exists but is an alias we overwrite it, otherwise we return
Command conflict = knownCommands.get(label);
if (conflict != null && conflict.getLabel().equals(label)) {
return false;
}
if (!isAlias) {
command.setLabel(label);
}
knownCommands.put(label, command);
return registered;
}
/**
* {@inheritDoc}
*/
public boolean dispatch(CommandSender sender, String commandLine) throws CommandException {
String[] args = PATTERN_ON_SPACE.split(commandLine);
if (args.length == 0) {
return false;
}
String sentCommandLabel = args[0].toLowerCase();
Command target = getCommand(sentCommandLabel);
if (target == null) {
return false;
}
try {
target.timings.startTiming(); // Spigot
// Note: we don't return the result of target.execute as thats success / failure, we return handled (true) or not handled (false)
target.execute(sender, sentCommandLabel, Arrays_copyOfRange(args, 1, args.length));
target.timings.stopTiming(); // Spigot
} catch (CommandException ex) {
target.timings.stopTiming(); // Spigot
throw ex;
} catch (Throwable ex) {
target.timings.stopTiming(); // Spigot
throw new CommandException("Unhandled exception executing '" + commandLine + "' in " + target, ex);
}
// return true as command was handled
return true;
}
public synchronized void clearCommands() {
for (Map.Entry entry : knownCommands.entrySet()) {
entry.getValue().unregister(this);
}
knownCommands.clear();
setDefaultCommands();
}
public Command getCommand(String name) {
Command target = knownCommands.get(name.toLowerCase());
return target;
}
public List tabComplete(CommandSender sender, String cmdLine) {
Validate.notNull(sender, "Sender cannot be null");
Validate.notNull(cmdLine, "Command line cannot null");
int spaceIndex = cmdLine.indexOf(' ');
if (spaceIndex == -1) {
ArrayList completions = new ArrayList();
Map knownCommands = this.knownCommands;
final String prefix = (sender instanceof Player ? "/" : "");
for (Map.Entry commandEntry : knownCommands.entrySet()) {
Command command = commandEntry.getValue();
if (!command.testPermissionSilent(sender)) {
continue;
}
String name = commandEntry.getKey(); // Use the alias, not command name
if (StringUtil.startsWithIgnoreCase(name, cmdLine)) {
completions.add(prefix + name);
}
}
Collections.sort(completions, String.CASE_INSENSITIVE_ORDER);
return completions;
}
String commandName = cmdLine.substring(0, spaceIndex);
Command target = getCommand(commandName);
if (target == null) {
return null;
}
if (!target.testPermissionSilent(sender)) {
return null;
}
String argLine = cmdLine.substring(spaceIndex + 1);
String[] args = PATTERN_ON_SPACE.split(argLine, -1);
try {
return target.tabComplete(sender, commandName, args);
} catch (CommandException ex) {
throw ex;
} catch (Throwable ex) {
throw new CommandException("Unhandled exception executing tab-completer for '" + cmdLine + "' in " + target, ex);
}
}
public Collection getCommands() {
return Collections.unmodifiableCollection(knownCommands.values());
}
public void registerServerAliases() {
Map values = server.getCommandAliases();
for (String alias : values.keySet()) {
if (alias.contains(":") || alias.contains(" ")) {
server.getLogger().warning("Could not register alias " + alias + " because it contains illegal characters");
continue;
}
String[] commandStrings = values.get(alias);
List targets = new ArrayList();
StringBuilder bad = new StringBuilder();
for (String commandString : commandStrings) {
String[] commandArgs = commandString.split(" ");
Command command = getCommand(commandArgs[0]);
if (command == null) {
if (bad.length() > 0) {
bad.append(", ");
}
bad.append(commandString);
} else {
targets.add(commandString);
}
}
if (bad.length() > 0) {
server.getLogger().warning("Could not register alias " + alias + " because it contains commands that do not exist: " + bad);
continue;
}
// We register these as commands so they have absolute priority.
if (targets.size() > 0) {
knownCommands.put(alias.toLowerCase(), new FormattedCommandAlias(alias.toLowerCase(), targets.toArray(new String[targets.size()])));
} else {
knownCommands.remove(alias.toLowerCase());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy