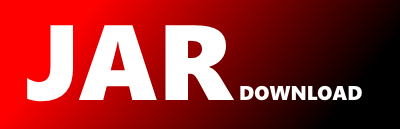
org.bukkit.command.defaults.OpCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of chest-server Show documentation
Show all versions of chest-server Show documentation
A spigot fork to kotlin structure and news.
The newest version!
package org.bukkit.command.defaults;
import com.google.common.collect.ImmutableList;
import org.apache.commons.lang.Validate;
import org.bukkit.Bukkit;
import org.bukkit.ChatColor;
import org.bukkit.OfflinePlayer;
import org.bukkit.command.Command;
import org.bukkit.command.CommandSender;
import org.bukkit.entity.Player;
import org.bukkit.util.StringUtil;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
@Deprecated
public class OpCommand extends VanillaCommand {
public OpCommand() {
super("op");
this.description = "Gives the specified player operator status";
this.usageMessage = "/op ";
this.setPermission("bukkit.command.op.give");
}
@Override
public boolean execute(CommandSender sender, String currentAlias, String[] args) {
if (!testPermission(sender)) return true;
if (args.length != 1 || args[0].length() == 0) {
sender.sendMessage(ChatColor.RED + "Usage: " + usageMessage);
return false;
}
OfflinePlayer player = Bukkit.getOfflinePlayer(args[0]);
player.setOp(true);
Command.broadcastCommandMessage(sender, "Opped " + args[0]);
return true;
}
@Override
public List tabComplete(CommandSender sender, String alias, String[] args) throws IllegalArgumentException {
Validate.notNull(sender, "Sender cannot be null");
Validate.notNull(args, "Arguments cannot be null");
Validate.notNull(alias, "Alias cannot be null");
if (args.length == 1) {
if (!(sender instanceof Player)) {
return ImmutableList.of();
}
String lastWord = args[0];
if (lastWord.length() == 0) {
return ImmutableList.of();
}
Player senderPlayer = (Player) sender;
ArrayList matchedPlayers = new ArrayList();
for (Player player : sender.getServer().getOnlinePlayers()) {
String name = player.getName();
if (!senderPlayer.canSee(player) || player.isOp()) {
continue;
}
if (StringUtil.startsWithIgnoreCase(name, lastWord)) {
matchedPlayers.add(name);
}
}
Collections.sort(matchedPlayers, String.CASE_INSENSITIVE_ORDER);
return matchedPlayers;
}
return ImmutableList.of();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy