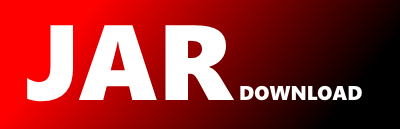
org.bukkit.craftbukkit.inventory.CraftShapelessRecipe Maven / Gradle / Ivy
package org.bukkit.craftbukkit.inventory;
import net.minecraft.server.CraftingManager;
import net.minecraft.server.ShapelessRecipes;
import org.bukkit.craftbukkit.util.CraftMagicNumbers;
import org.bukkit.inventory.ItemStack;
import org.bukkit.inventory.ShapelessRecipe;
import java.util.List;
public class CraftShapelessRecipe extends ShapelessRecipe implements CraftRecipe {
// TODO: Could eventually use this to add a matches() method or some such
private ShapelessRecipes recipe;
public CraftShapelessRecipe(ItemStack result) {
super(result);
}
public CraftShapelessRecipe(ItemStack result, ShapelessRecipes recipe) {
this(result);
this.recipe = recipe;
}
public static CraftShapelessRecipe fromBukkitRecipe(ShapelessRecipe recipe) {
if (recipe instanceof CraftShapelessRecipe) {
return (CraftShapelessRecipe) recipe;
}
CraftShapelessRecipe ret = new CraftShapelessRecipe(recipe.getResult());
for (ItemStack ingred : recipe.getIngredientList()) {
ret.addIngredient(ingred.getType(), ingred.getDurability());
}
return ret;
}
public void addToCraftingManager() {
List ingred = this.getIngredientList();
Object[] data = new Object[ingred.size()];
int i = 0;
for (ItemStack mdata : ingred) {
int id = mdata.getTypeId();
short dmg = mdata.getDurability();
data[i] = new net.minecraft.server.ItemStack(CraftMagicNumbers.getItem(id), 1, dmg);
i++;
}
CraftingManager.getInstance().registerShapelessRecipe(CraftItemStack.asNMSCopy(this.getResult()), data);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy