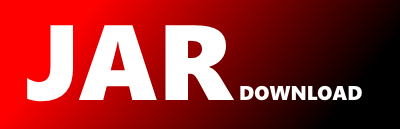
org.bukkit.event.server.ServerListPingEvent Maven / Gradle / Ivy
package org.bukkit.event.server;
import org.apache.commons.lang.Validate;
import org.bukkit.entity.Player;
import org.bukkit.event.HandlerList;
import org.bukkit.util.CachedServerIcon;
import java.net.InetAddress;
import java.util.Iterator;
/**
* Called when a server list ping is coming in. Displayed players can be
* checked and removed by {@link #iterator() iterating} over this event.
*/
public class ServerListPingEvent extends ServerEvent implements Iterable {
private static final int MAGIC_PLAYER_COUNT = Integer.MIN_VALUE;
private static final HandlerList handlers = new HandlerList();
private final InetAddress address;
private final int numPlayers;
private String motd;
private int maxPlayers;
public ServerListPingEvent(final InetAddress address, final String motd, final int numPlayers, final int maxPlayers) {
Validate.isTrue(numPlayers >= 0, "Cannot have negative number of players online", numPlayers);
this.address = address;
this.motd = motd;
this.numPlayers = numPlayers;
this.maxPlayers = maxPlayers;
}
/**
* This constructor is intended for implementations that provide the
* {@link #iterator()} method, thus provided the {@link #getNumPlayers()}
* count.
*
* @param address the address of the pinger
* @param motd the message of the day
* @param maxPlayers the max number of players
*/
protected ServerListPingEvent(final InetAddress address, final String motd, final int maxPlayers) {
this.numPlayers = MAGIC_PLAYER_COUNT;
this.address = address;
this.motd = motd;
this.maxPlayers = maxPlayers;
}
public static HandlerList getHandlerList() {
return handlers;
}
/**
* Get the address the ping is coming from.
*
* @return the address
*/
public InetAddress getAddress() {
return address;
}
/**
* Get the message of the day message.
*
* @return the message of the day
*/
public String getMotd() {
return motd;
}
/**
* Change the message of the day message.
*
* @param motd the message of the day
*/
public void setMotd(String motd) {
this.motd = motd;
}
/**
* Get the number of players sent.
*
* @return the number of players
*/
public int getNumPlayers() {
int numPlayers = this.numPlayers;
if (numPlayers == MAGIC_PLAYER_COUNT) {
numPlayers = 0;
for (@SuppressWarnings("unused") final Player player : this) {
numPlayers++;
}
}
return numPlayers;
}
/**
* Get the maximum number of players sent.
*
* @return the maximum number of players
*/
public int getMaxPlayers() {
return maxPlayers;
}
/**
* Set the maximum number of players sent.
*
* @param maxPlayers the maximum number of player
*/
public void setMaxPlayers(int maxPlayers) {
this.maxPlayers = maxPlayers;
}
/**
* Sets the server-icon sent to the client.
*
* @param icon the icon to send to the client
* @throws IllegalArgumentException if the {@link CachedServerIcon} is not
* created by the caller of this event; null may be accepted for some
* implementations
* @throws UnsupportedOperationException if the caller of this event does
* not support setting the server icon
*/
public void setServerIcon(CachedServerIcon icon) throws IllegalArgumentException, UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@Override
public HandlerList getHandlers() {
return handlers;
}
/**
* {@inheritDoc}
*
* Calling the {@link Iterator#remove()} method will force that particular
* player to not be displayed on the player list, decrease the size
* returned by {@link #getNumPlayers()}, and will not be returned again by
* any new iterator.
*
* @throws UnsupportedOperationException if the caller of this event does
* not support removing players
*/
@Override
public Iterator iterator() throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy