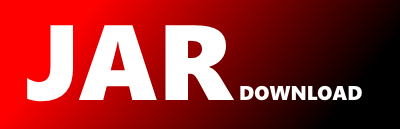
net.minecraft.server.BaseBlockPosition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package net.minecraft.server;
import com.google.common.base.Objects;
import org.jetbrains.annotations.NotNull;
public class BaseBlockPosition implements Comparable {
public static final BaseBlockPosition ZERO = new BaseBlockPosition(0, 0, 0);
public final int a;
public final int c;
public final int d;
public BaseBlockPosition(int i, int j, int k) {
this.a = i;
this.c = j;
this.d = k;
}
public BaseBlockPosition(double d0, double d1, double d2) {
this(MathHelper.floor(d0), MathHelper.floor(d1), MathHelper.floor(d2));
}
public boolean equals(Object object) {
if (this == object) {
return true;
} else if (!(object instanceof BaseBlockPosition)) {
return false;
} else {
BaseBlockPosition baseblockposition = (BaseBlockPosition) object;
return this.getX() == baseblockposition.getX() && (this.getY() == baseblockposition.getY() && this.getZ() == baseblockposition.getZ());
}
}
public int hashCode() {
return (this.getY() + this.getZ() * 31) * 31 + this.getX();
}
public int g(BaseBlockPosition baseblockposition) {
return this.getY() == baseblockposition.getY() ? (this.getZ() == baseblockposition.getZ() ? this.getX() - baseblockposition.getX() : this.getZ() - baseblockposition.getZ()) : this.getY() - baseblockposition.getY();
}
public int getX() {
return this.a;
}
public int getY() {
return this.c;
}
public int getZ() {
return this.d;
}
public BaseBlockPosition d(BaseBlockPosition baseblockposition) {
return new BaseBlockPosition(this.getY() * baseblockposition.getZ() - this.getZ() * baseblockposition.getY(), this.getZ() * baseblockposition.getX() - this.getX() * baseblockposition.getZ(), this.getX() * baseblockposition.getY() - this.getY() * baseblockposition.getX());
}
public double c(double d0, double d1, double d2) {
double d3 = (double) this.getX() - d0;
double d4 = (double) this.getY() - d1;
double d5 = (double) this.getZ() - d2;
return d3 * d3 + d4 * d4 + d5 * d5;
}
public double d(double d0, double d1, double d2) {
double d3 = (double) this.getX() + 0.5D - d0;
double d4 = (double) this.getY() + 0.5D - d1;
double d5 = (double) this.getZ() + 0.5D - d2;
return d3 * d3 + d4 * d4 + d5 * d5;
}
public double i(BaseBlockPosition baseblockposition) {
return this.c(baseblockposition.getX(), baseblockposition.getY(), baseblockposition.getZ());
}
public String toString() {
return Objects.toStringHelper(this).add("x", this.getX()).add("y", this.getY()).add("z", this.getZ()).toString();
}
public int compareTo(@NotNull BaseBlockPosition object) {
return this.g(object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy