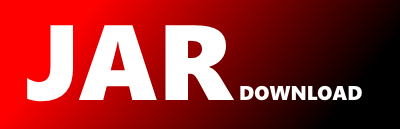
net.minecraft.server.InventoryCrafting Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package net.minecraft.server;
// CraftBukkit start
import org.bukkit.craftbukkit.entity.CraftHumanEntity;
import org.bukkit.entity.HumanEntity;
import org.bukkit.event.inventory.InventoryType;
import java.util.List;
// CraftBukkit end
public class InventoryCrafting implements IInventory {
private final ItemStack[] items;
private final int b;
private final int c;
private final Container d;
// CraftBukkit start - add fields
public List transaction = new java.util.ArrayList();
public IRecipe currentRecipe;
public IInventory resultInventory;
private EntityHuman owner;
private int maxStack = MAX_STACK;
public InventoryCrafting(Container container, int i, int j, EntityHuman player) {
this(container, i, j);
this.owner = player;
}
public InventoryCrafting(Container container, int i, int j) {
int k = i * j;
this.items = new ItemStack[k];
this.d = container;
this.b = i;
this.c = j;
}
public ItemStack[] getContents() {
return this.items;
}
public void onOpen(CraftHumanEntity who) {
transaction.add(who);
}
public InventoryType getInvType() {
return items.length == 4 ? InventoryType.CRAFTING : InventoryType.WORKBENCH;
}
public void onClose(CraftHumanEntity who) {
transaction.remove(who);
}
public List getViewers() {
return transaction;
}
public org.bukkit.inventory.InventoryHolder getOwner() {
return (owner == null) ? null : owner.getBukkitEntity();
}
// CraftBukkit end
public int getSize() {
return this.items.length;
}
public ItemStack getItem(int i) {
return i >= this.getSize() ? null : this.items[i];
}
public ItemStack c(int i, int j) {
return i >= 0 && i < this.b && j >= 0 && j <= this.c ? this.getItem(i + j * this.b) : null;
}
public String getName() {
return "container.crafting";
}
public boolean hasCustomName() {
return false;
}
public IChatBaseComponent getScoreboardDisplayName() {
return this.hasCustomName() ? new ChatComponentText(this.getName()) : new ChatMessage(this.getName(), new Object[0]);
}
public ItemStack splitWithoutUpdate(int i) {
if (this.items[i] != null) {
ItemStack itemstack = this.items[i];
this.items[i] = null;
return itemstack;
} else {
return null;
}
}
public ItemStack splitStack(int i, int j) {
if (this.items[i] != null) {
ItemStack itemstack;
if (this.items[i].count <= j) {
itemstack = this.items[i];
this.items[i] = null;
this.d.a(this);
return itemstack;
} else {
itemstack = this.items[i].cloneAndSubtract(j);
if (this.items[i].count == 0) {
this.items[i] = null;
}
this.d.a(this);
return itemstack;
}
} else {
return null;
}
}
public void setItem(int i, ItemStack itemstack) {
this.items[i] = itemstack;
this.d.a(this);
}
public int getMaxStackSize() {
return 64;
}
public void setMaxStackSize(int size) {
maxStack = size;
resultInventory.setMaxStackSize(size);
}
public void update() {
}
public boolean a(EntityHuman entityhuman) {
return true;
}
public void startOpen(EntityHuman entityhuman) {
}
public void closeContainer(EntityHuman entityhuman) {
}
public boolean b(int i, ItemStack itemstack) {
return true;
}
public int getProperty(int i) {
return 0;
}
public void b(int i, int j) {
}
public int g() {
return 0;
}
public void l() {
for (int i = 0; i < this.items.length; ++i) {
this.items[i] = null;
}
}
public int h() {
return this.c;
}
public int i() {
return this.b;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy