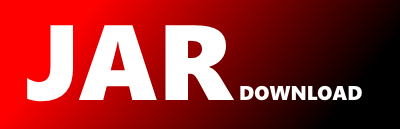
net.minecraft.server.Scoreboard Maven / Gradle / Ivy
package net.minecraft.server;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import java.util.*;
public class Scoreboard {
private final Map objectivesByName = Maps.newHashMap();
private final Map> objectivesByCriteria = Maps.newHashMap();
private final Map> playerScores = Maps.newHashMap();
private final ScoreboardObjective[] displaySlots = new ScoreboardObjective[19];
private final Map teamsByName = Maps.newHashMap();
private final Map teamsByPlayer = Maps.newHashMap();
private static String[] g = null;
public Scoreboard() {
}
public ScoreboardObjective getObjective(String s) {
return this.objectivesByName.get(s);
}
public ScoreboardObjective registerObjective(String s, IScoreboardCriteria iscoreboardcriteria) {
if (s.length() > 96) {
throw new IllegalArgumentException("The objective name '" + s + "' is too long!");
} else {
ScoreboardObjective scoreboardobjective = this.getObjective(s);
if (scoreboardobjective != null) {
throw new IllegalArgumentException("An objective with the name '" + s + "' already exists!");
} else {
scoreboardobjective = new ScoreboardObjective(this, s, iscoreboardcriteria);
List object = this.objectivesByCriteria.computeIfAbsent(iscoreboardcriteria, k -> Lists.newArrayList());
object.add(scoreboardobjective);
this.objectivesByName.put(s, scoreboardobjective);
this.handleObjectiveAdded(scoreboardobjective);
return scoreboardobjective;
}
}
}
public Collection getObjectivesForCriteria(IScoreboardCriteria iscoreboardcriteria) {
Collection collection = this.objectivesByCriteria.get(iscoreboardcriteria);
return collection == null ? Lists.newArrayList() : Lists.newArrayList(collection);
}
public boolean b(String s, ScoreboardObjective scoreboardobjective) {
Map map = this.playerScores.get(s);
if (map == null) {
return false;
} else {
ScoreboardScore scoreboardscore = (ScoreboardScore) map.get(scoreboardobjective);
return scoreboardscore != null;
}
}
public ScoreboardScore getPlayerScoreForObjective(String s, ScoreboardObjective scoreboardobjective) {
if (s.length() > 120) {
throw new IllegalArgumentException("The player name '" + s + "' is too long!");
} else {
Map object = this.playerScores.computeIfAbsent(s, k -> Maps.newHashMap());
ScoreboardScore scoreboardscore = (ScoreboardScore) ((Map, ?>) object).get(scoreboardobjective);
if (scoreboardscore == null) {
scoreboardscore = new ScoreboardScore(this, scoreboardobjective, s);
((Map) object).put(scoreboardobjective, scoreboardscore);
}
return scoreboardscore;
}
}
public Collection getScoresForObjective(ScoreboardObjective scoreboardobjective) {
ArrayList arraylist = Lists.newArrayList();
for (Map scoreboardObjectiveScoreboardScoreMap : this.playerScores.values()) {
ScoreboardScore scoreboardscore = (ScoreboardScore) ((Map, ?>) scoreboardObjectiveScoreboardScoreMap).get(scoreboardobjective);
if (scoreboardscore != null) {
arraylist.add(scoreboardscore);
}
}
arraylist.sort(ScoreboardScore.a);
return arraylist;
}
public Collection getObjectives() {
return this.objectivesByName.values();
}
public Collection getPlayers() {
return this.playerScores.keySet();
}
public void resetPlayerScores(String s, ScoreboardObjective scoreboardobjective) {
Map map;
if (scoreboardobjective == null) {
map = this.playerScores.remove(s);
if (map != null) {
this.handlePlayerRemoved(s);
}
} else {
map = this.playerScores.get(s);
if (map != null) {
ScoreboardScore scoreboardscore = (ScoreboardScore) map.remove(scoreboardobjective);
if (map.size() < 1) {
Map map1 = this.playerScores.remove(s);
if (map1 != null) {
this.handlePlayerRemoved(s);
}
} else if (scoreboardscore != null) {
this.a(s, scoreboardobjective);
}
}
}
}
public Collection getScores() {
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy