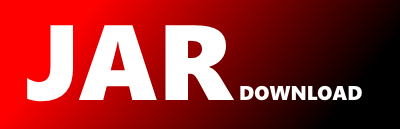
org.bukkit.command.defaults.DefaultGameModeCommand Maven / Gradle / Ivy
package org.bukkit.command.defaults;
import com.google.common.collect.ImmutableList;
import org.apache.commons.lang.Validate;
import org.bukkit.Bukkit;
import org.bukkit.GameMode;
import org.bukkit.command.Command;
import org.bukkit.command.CommandSender;
import org.bukkit.util.StringUtil;
import java.util.ArrayList;
import java.util.List;
@Deprecated
public class DefaultGameModeCommand extends VanillaCommand {
private static final List GAMEMODE_NAMES = ImmutableList.of("adventure", "creative", "survival");
public DefaultGameModeCommand() {
super("defaultgamemode");
this.description = "Set the default gamemode";
this.usageMessage = "/defaultgamemode ";
this.setPermission("bukkit.command.defaultgamemode");
}
@Override
public boolean execute(CommandSender sender, String commandLabel, String[] args) {
if (!testPermission(sender)) return true;
if (args.length == 0) {
sender.sendMessage("Usage: " + usageMessage);
return false;
}
String modeArg = args[0];
int value = -1;
try {
value = Integer.parseInt(modeArg);
} catch (NumberFormatException ex) {
}
GameMode mode = GameMode.getByValue(value);
if (mode == null) {
if (modeArg.equalsIgnoreCase("creative") || modeArg.equalsIgnoreCase("c")) {
mode = GameMode.CREATIVE;
} else if (modeArg.equalsIgnoreCase("adventure") || modeArg.equalsIgnoreCase("a")) {
mode = GameMode.ADVENTURE;
} else {
mode = GameMode.SURVIVAL;
}
}
Bukkit.getServer().setDefaultGameMode(mode);
Command.broadcastCommandMessage(sender, "Default game mode set to " + mode.toString().toLowerCase());
return true;
}
@Override
public List tabComplete(CommandSender sender, String alias, String[] args) {
Validate.notNull(sender, "Sender cannot be null");
Validate.notNull(args, "Arguments cannot be null");
Validate.notNull(alias, "Alias cannot be null");
if (args.length == 1) {
return StringUtil.copyPartialMatches(args[0], GAMEMODE_NAMES, new ArrayList(GAMEMODE_NAMES.size()));
}
return ImmutableList.of();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy