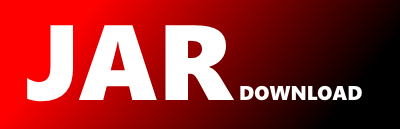
org.bukkit.event.entity.EntityRegainHealthEvent Maven / Gradle / Ivy
package org.bukkit.event.entity;
import org.bukkit.entity.Entity;
import org.bukkit.event.Cancellable;
import org.bukkit.event.HandlerList;
import org.bukkit.util.NumberConversions;
/**
* Stores data for health-regain events
*/
public class EntityRegainHealthEvent extends EntityEvent implements Cancellable {
private static final HandlerList handlers = new HandlerList();
private final RegainReason regainReason;
private boolean cancelled;
private double amount;
@Deprecated
public EntityRegainHealthEvent(final Entity entity, final int amount, final RegainReason regainReason) {
this(entity, (double) amount, regainReason);
}
public EntityRegainHealthEvent(final Entity entity, final double amount, final RegainReason regainReason) {
super(entity);
this.amount = amount;
this.regainReason = regainReason;
}
public static HandlerList getHandlerList() {
return handlers;
}
/**
* Gets the amount of regained health
*
* @return The amount of health regained
*/
public double getAmount() {
return amount;
}
/**
* Sets the amount of regained health
*
* @param amount the amount of health the entity will regain
*/
public void setAmount(double amount) {
this.amount = amount;
}
/**
* This method exists for legacy reasons to provide backwards
* compatibility. It will not exist at runtime and should not be used
* under any circumstances.
*
* @return the (rounded) amount regained
*/
@Deprecated
public int _INVALID_getAmount() {
return NumberConversions.ceil(getAmount());
}
/**
* This method exists for legacy reasons to provide backwards
* compatibility. It will not exist at runtime and should not be used
* under any circumstances.
*
* @param amount the amount that will be regained
*/
@Deprecated
public void _INVALID_setAmount(int amount) {
setAmount(amount);
}
@Override
public boolean isCancelled() {
return cancelled;
}
@Override
public void setCancelled(boolean cancel) {
cancelled = cancel;
}
/**
* Gets the reason for why the entity is regaining health
*
* @return A RegainReason detailing the reason for the entity regaining
* health
*/
public RegainReason getRegainReason() {
return regainReason;
}
@Override
public HandlerList getHandlers() {
return handlers;
}
/**
* An enum to specify the type of health regaining that is occurring
*/
public enum RegainReason {
/**
* When a player regains health from regenerating due to Peaceful mode
* (difficulty=0)
*/
REGEN,
/**
* When a player regains health from regenerating due to their hunger
* being satisfied
*/
SATIATED,
/**
* When a player regains health from eating consumables
*/
EATING,
/**
* When an ender dragon regains health from an ender crystal
*/
ENDER_CRYSTAL,
/**
* When a player is healed by a potion or spell
*/
MAGIC,
/**
* When a player is healed over time by a potion or spell
*/
MAGIC_REGEN,
/**
* When a wither is filling its health during spawning
*/
WITHER_SPAWN,
/**
* When an entity is damaged by the Wither potion effect
*/
WITHER,
/**
* Any other reason not covered by the reasons above
*/
CUSTOM
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy