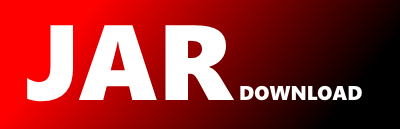
org.bukkit.event.player.PlayerChatTabCompleteEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package org.bukkit.event.player;
import org.apache.commons.lang.Validate;
import org.bukkit.entity.Player;
import org.bukkit.event.Cancellable;
import org.bukkit.event.HandlerList;
import java.util.Collection;
/**
* Called when a player attempts to tab-complete a chat message.
*/
public class PlayerChatTabCompleteEvent extends PlayerEvent implements Cancellable {
private static final HandlerList handlers = new HandlerList();
private final String message;
private final String lastToken;
private final Collection completions;
private boolean cancelled = false;
public PlayerChatTabCompleteEvent(final Player who, final String message, final Collection completions) {
super(who);
Validate.notNull(message, "Message cannot be null");
Validate.notNull(completions, "Completions cannot be null");
this.message = message;
int i = message.lastIndexOf(' ');
if (i < 0) {
this.lastToken = message;
} else {
this.lastToken = message.substring(i + 1);
}
this.completions = completions;
}
public static HandlerList getHandlerList() {
return handlers;
}
/**
* Gets the chat message being tab-completed.
*
* @return the chat message
*/
public String getChatMessage() {
return message;
}
/**
* Gets the last 'token' of the message being tab-completed.
*
* The token is the substring starting with the character after the last
* space in the message.
*
* @return The last token for the chat message
*/
public String getLastToken() {
return lastToken;
}
/**
* This is the collection of completions for this event.
*
* @return the current completions
*/
public Collection getTabCompletions() {
return completions;
}
@Override
public HandlerList getHandlers() {
return handlers;
}
@Override
public boolean isCancelled() {
return cancelled;
}
@Override
public void setCancelled(boolean cancel) {
this.cancelled = cancel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy