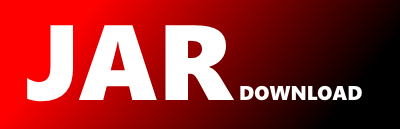
walkmc.BoundingBox.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package walkmc
import kotlinx.serialization.*
import kotlinx.serialization.descriptors.*
import kotlinx.serialization.encoding.*
import net.minecraft.server.*
import org.bukkit.*
import org.bukkit.World
import org.bukkit.block.Block
import org.bukkit.entity.Entity
/**
* A bounding box represents a hit box used for some things. This is similar to [AxisAlignedBB].
*/
@Serializable(BoundingBoxSerializer::class)
data class BoundingBox(
var minX: Double,
var minY: Double,
var minZ: Double,
var maxX: Double,
var maxY: Double,
var maxZ: Double,
) {
constructor(min: Block, max: Block) : this(min.location, max.location)
constructor(min: Entity, max: Entity) : this(min.location, max.location)
constructor(axis: AxisAlignedBB) : this(axis.a, axis.b, axis.c, axis.d, axis.e, axis.f)
constructor(min: Location, max: Location) : this(min.x, min.y, min.z, max.x, max.y, max.z)
/**
* Grows this bounding box to the amount of radius.
*/
fun grow(radius: Int): BoundingBox = apply {
minX -= radius
minY -= radius
minZ -= radius
maxX += radius
maxY += radius
maxZ += radius
}
/**
* Shrinks this bounding box to the amount of radius.
*/
fun shrink(radius: Int): BoundingBox = apply {
minX += radius
minY += radius
minZ += radius
maxX -= radius
maxY -= radius
maxZ -= radius
}
/**
* Verifies if a box is collind with this box.
*/
fun isColliding(other: BoundingBox): Boolean =
isCollidingX(other) || isCollidingY(other) || isCollidingZ(other)
/**
* Verifies if the X of a box is collind with X of this box.
*/
fun isCollidingX(other: BoundingBox): Boolean = other.maxX > minX && other.minX < maxX
/**
* Verifies if the Y of a box is collind with Y of this box.
*/
fun isCollidingY(other: BoundingBox): Boolean = other.maxY > minY && other.minY < maxY
/**
* Verifies if the Z of a box is collind with Z of this box.
*/
fun isCollidingZ(other: BoundingBox): Boolean = other.maxZ > minZ && other.minZ < maxZ
/**
* Verifies if a location is inside this box.
*/
fun isInside(loc: Location): Boolean = loc.x in minX..maxX && loc.y in minY..maxY && loc.z in minZ..maxZ
/**
* Verifies if a block is inside this box.
*/
fun isInside(block: Block): Boolean = isInside(block.location)
/**
* Verifies if an entity is inside this box.
*/
fun isInside(entity: Entity): Boolean = isInside(entity.location)
/**
* Converts this box to a [AxisAlignedBB].
*/
fun toAxis(): AxisAlignedBB = AxisAlignedBB(minX, minY, minZ, maxX, maxY, maxZ)
/**
* Converts this box to a [StructureBox].
*/
fun toStructureBox(world: World) = StructureBox(
world,
minX.toInt(),
minY.toInt(),
minZ.toInt(),
maxX.toInt(),
maxY.toInt(),
maxZ.toInt()
)
override fun toString(): String = "$minX:$minY:$minZ:$maxX:$maxY:$maxZ"
companion object {
/**
* Converts a string to a bounding box.
*/
fun from(string: String): BoundingBox {
val split = string.split(':', limit = 6)
return BoundingBox(
split[0].toDouble(),
split[1].toDouble(),
split[2].toDouble(),
split[3].toDouble(),
split[4].toDouble(),
split[5].toDouble(),
)
}
}
}
/**
* A serializer for serializing [BoundingBox].
*/
object BoundingBoxSerializer : KSerializer {
override val descriptor = PrimitiveSerialDescriptor("BoundingBox", PrimitiveKind.STRING)
override fun deserialize(decoder: Decoder): BoundingBox {
return BoundingBox.from(decoder.decodeString())
}
override fun serialize(encoder: Encoder, value: BoundingBox) {
encoder.encodeString(value.toString())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy