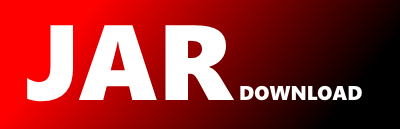
walkmc.Coordinate.kt Maven / Gradle / Ivy
package walkmc
import kotlinx.serialization.*
import org.bukkit.*
import org.bukkit.util.*
/**
* A coordinate represents the **x**, **y** and **z** of a location.
* This is used to store certain data without needing the world.
*/
@Serializable
data class Coordinate(var x: Double, var y: Double, var z: Double) {
/**
* Converts this coordinate to location by the specified world.
*/
fun toLocation(world: World): Location {
return Location(world, x, y, z)
}
/**
* Converts this coordinate to a vector.
*/
fun toVector(): Vector {
return Vector(x, y, z)
}
/**
* Converts this coordinate to a block vector.
*/
fun toBlockVector(): BlockVector {
return BlockVector(x, y, z)
}
override fun toString(): String {
return "$x|$y|$z"
}
companion object {
/**
* Creates a new coordinate by the specified x, y and z.
*/
fun of(x: Number, y: Number, z: Number): Coordinate {
return Coordinate(x.toDouble(), y.toDouble(), z.toDouble())
}
/**
* Parses a new coordinate by the specified string.
*/
fun from(string: String): Coordinate {
val split = string.split('|', limit = 3)
return Coordinate(split[0].toDouble(), split[1].toDouble(), split[2].toDouble())
}
/**
* Converts the specified location to a coordinate.
*/
fun from(location: Location): Coordinate {
return Coordinate(location.x, location.y, location.z)
}
/**
* Converts the specified vector to a coordinate.
*/
fun from(vector: Vector): Coordinate {
return Coordinate(vector.x, vector.y, vector.z)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy