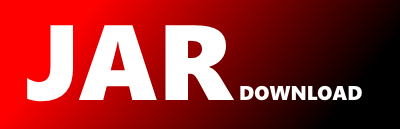
walkmc.Description.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc
import kotlinx.serialization.*
import org.bukkit.inventory.*
import walkmc.extensions.*
/**
* Represents a description that's describes some information of anything.
* Also provides convenient functions to implement the description in other classes.
*/
@Serializable
@JvmInline
value class Description(val value: List) {
constructor(str: String) : this(str.lines())
}
/**
* Implements the specified [description] on the lore of this item.
*
* @param atStart where the description is implemented in the start of the lore.
* A value of true represents the start of the lore. A value of false
* represents the end of the lore.
*/
fun ItemStack.describes(description: Description, atStart: Boolean) {
applyMeta {
lore.describes(description, atStart)
}
}
/**
* Implements the specified [description] on the lore of this item.
*
* @param index the start index to implement the description in the lore.
*/
fun ItemStack.describes(description: Description, index: Int = 0) {
applyMeta {
lore.describes(description, index)
}
}
/**
* Implements the specified [description] on this list
*
* @param atStart where the description is implemented in the start of the list.
* A value of true represents the start of the list. A value of false
* represents the end of the list.
*/
fun MutableList.describes(description: Description, atStart: Boolean) {
if (atStart) addAll(0, description.value) else addAll(description.value)
}
/**
* Implements the specified [description] on the this list
*
* @param index the start index to implement the description in the list.
*/
inline fun MutableList.describes(description: Description, index: Int = 0) {
addAll(index, description.value)
}
/**
* Implements the specified [description] on the end of this list
*/
inline operator fun MutableList.plusAssign(description: Description) {
addAll(description.value)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy