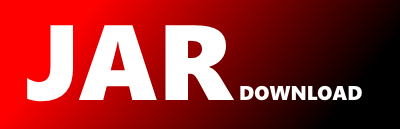
walkmc.Downloader.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package walkmc
import walkmc.extensions.*
import walkmc.extensions.numbers.*
import java.io.*
import java.net.*
/**
* Represents a downloader used to download files from a URL.
*/
object Downloader {
/**
* Downloads the file at specified url and returns the file.
*/
fun download(url: URL, print: Boolean = false): File {
val connection = url.openConnection() as HttpURLConnection
val name = url.file.substring(url.file.lastIndexOf('/') + 1)
val file = kotlin.io.path.createTempFile(name).toFile()
if (print)
println("Downloading $name...")
connection.inputStream.buffered().use { input ->
file.outputStream().buffered().use { output ->
val copy = (input.copyTo(output) / 1024).spaced()
if (print)
println("Downloaded $name with $copy kb size")
}
}
return file
}
/**
* Downloads the file at specified url and implements inside of the specified folder
* and returns the file.
*/
fun download(url: URL, to: File, print: Boolean = false): File {
val name = url.file.substring(url.file.lastIndexOf('/') + 1)
val file = file(to, name)
if (file.exists()) return file else file.create()
val connection = url.openConnection() as HttpURLConnection
if (print)
println("Downloading $name...")
connection.inputStream.buffered().use { input ->
file.outputStream().buffered().use { output ->
val copy = (input.copyTo(output) / 1024).spaced()
if (print)
println("Downloaded $name with $copy kb size")
}
}
return file
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy