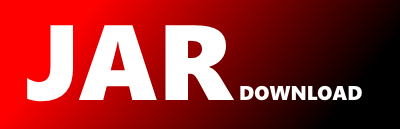
walkmc.NamespacedKey.kt Maven / Gradle / Ivy
package walkmc
import kotlinx.serialization.*
import kotlinx.serialization.descriptors.*
import kotlinx.serialization.encoding.*
/**
* A namespaced key is a identifier key with a namespace and a key.
* This is commonly used to store objects that need a id.
* All [NamespacedKey] will be formated as lowercase.
*/
@Serializable(NamespacedKeySerializer::class)
data class NamespacedKey internal constructor(
val namespace: String,
val key: String,
) : Comparable {
companion object {
/**
* Constructs a new namespaced key with the specified namespace and key.
*/
fun of(namespace: String, key: String): NamespacedKey =
NamespacedKey(namespace.lowercase(), key.lowercase())
/**
* Converts the specified string to a namespaced key.
*/
fun from(string: String): NamespacedKey {
val split = string.split(':', limit = 2)
return of(split[0], split[1])
}
}
override fun toString(): String {
return "$namespace:$key"
}
override fun compareTo(other: NamespacedKey): Int {
return toString().compareTo(other.toString())
}
}
/**
* Creates a new [NamespacedKey] by [namespace] and [key].
*/
fun namespacedKeyOf(namespace: String, key: String) = NamespacedKey.of(namespace, key)
/**
* A serializer type used to serialize/deserialize [NamespacedKey].
*/
object NamespacedKeySerializer : KSerializer {
override val descriptor = PrimitiveSerialDescriptor("NamespacedKey", PrimitiveKind.STRING)
override fun deserialize(decoder: Decoder): NamespacedKey {
return NamespacedKey.from(decoder.decodeString())
}
override fun serialize(encoder: Encoder, value: NamespacedKey) {
encoder.encodeString(value.toString())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy