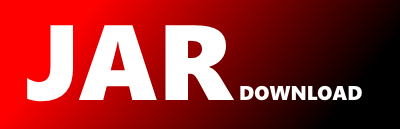
walkmc.Particle.kt Maven / Gradle / Ivy
package walkmc
import net.minecraft.server.*
import org.bukkit.*
import org.bukkit.entity.*
import walkmc.extensions.*
/**
* A particle enum used to send all particles.
*/
enum class Particle(val namespace: String, val id: Int) {
EXPLOSION_NORMAL("explode", 0),
EXPLOSION_LARGE("largeexplode", 1),
EXPLOSION_HUGE("hugeexplosion", 2),
FIREWORKS_SPARK("fireworksSpark", 3),
WATER_BUBBLE("bubble", 4),
WATER_SPLASH("splash", 5),
WATER_WAKE("wake", 6),
SUSPENDED("suspended", 7),
SUSPENDED_DEPTH("depthsuspend", 8),
CRIT("crit", 9),
CRIT_MAGIC("magicCrit", 10),
SMOKE_NORMAL("smoke", 11),
SMOKE_LARGE("largesmoke", 12),
SPELL("spell", 13),
SPELL_INSTANT("instantSpell", 14),
SPELL_MOB("mobSpell", 15),
SPELL_MOB_AMBIENT("mobSpellAmbient", 16),
SPELL_WITCH("witchMagic", 17),
DRIP_WATER("dripWater", 18),
DRIP_LAVA("dripLava", 19),
VILLAGER_ANGRY("angryVillager", 20),
VILLAGER_HAPPY("happyVillager", 21),
TOWN_AURA("townaura", 22),
NOTE("note", 23),
PORTAL("portal", 24),
ENCHANTMENT_TABLE("enchantmenttable", 25),
FLAME("flame", 26),
LAVA("lava", 27),
FOOTSTEP("footstep", 28),
CLOUD("cloud", 29),
REDSTONE("reddust", 30),
SNOWBALL("snowballpoof", 31),
SNOW_SHOVEL("snowshovel", 32),
SLIME("slime", 33),
HEART("heart", 34),
BARRIER("barrier", 35),
ITEM_CRACK("iconcrack_", 36),
BLOCK_CRACK("blockcrack_", 37),
BLOCK_DUST("blockdust_", 38),
WATER_DROP("droplet", 39),
ITEM_TAKE("take", 40),
MOB_APPEARANCE("mobappearance", 41);
/**
* Returns if this particle can have colors.
*/
val hasColor: Boolean
get() = id == 15 || id == 16 || id == 30
/**
* Returns if this particle must have a specified material data to be able to use.
*/
val mustHaveMaterial: Boolean
get() = id == 36 || id == 37 || id == 38 || id == 40
/**
* Plays this particle to only specified player in the specified location.
*/
fun play(
player: Player,
location: Location,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
vararg options: Int,
) = player.sendPacket(
PacketPlayOutWorldParticles(
toEnumParticle(), true, location.x.toFloat(), location.y.toFloat(), location.z.toFloat(),
offsetX, offsetY, offsetZ, speed, amount, *options
)
)
/**
* Plays this particle to all players of the location world in the specified location.
*/
fun play(
location: Location,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
vararg options: Int,
) {
val packet = PacketPlayOutWorldParticles(
toEnumParticle(), true, location.x.toFloat(), location.y.toFloat(), location.z.toFloat(),
offsetX, offsetY, offsetZ, speed, amount, *options
)
for (player in location.world.players) {
player.sendPacket(packet)
}
}
/**
* Plays this particle to all players in the specified location world
* filtered by the given [predicate].
*/
inline fun play(
location: Location,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
vararg options: Int,
predicate: (Player) -> Boolean
) {
val packet = PacketPlayOutWorldParticles(
toEnumParticle(), true, location.x.toFloat(), location.y.toFloat(), location.z.toFloat(),
offsetX, offsetY, offsetZ, speed, amount, *options
)
for (player in location.world.players) {
if (predicate(player)) {
player.sendPacket(packet)
}
}
}
/**
* Returns the NMS [EnumParticle] representation of this particle.
*/
fun toEnumParticle(): EnumParticle {
return EnumParticle.valueOf(name)
}
companion object {
/**
* Plays a coloured particle type with the specified [color] in the given location
* for only to the specified [player].
*
* @param player the unique player that's will be able to see the particle.
* @param color the color that will be displayed.
* @param location the location that the particle will spawn.
*/
fun playColoured(particle: Particle, player: Player, location: Location, color: Color) {
if (!particle.hasColor)
return
particle.play(player, location, 0, 1f, color.red / 255f, color.green / 255f, color.blue / 255f)
}
/**
* Plays a coloured particle type with the specified [color] in the given location
* for all players in the location world.
*
* @param color the color that will be displayed.
* @param location the location that the particle will spawn.
*/
fun playColoured(particle: Particle, location: Location, color: Color) {
if (!particle.hasColor)
return
particle.play(location, 0, 1f, color.red / 255f, color.green / 255f, color.blue / 255f)
}
/**
* Plays a coloured particle type with the specified [color] in the given location
* for all players in the location world filtered by [predicate].
*
* @param color the color that will be displayed.
* @param location the location that the particle will spawn.
*/
inline fun playColoured(
particle: Particle,
location: Location,
color: Color,
predicate: (Player) -> Boolean
) {
if (!particle.hasColor)
return
particle.play(location, 0, 1f, color.red / 255f, color.green / 255f, color.blue / 255f, predicate = predicate)
}
/**
* @see playColoured
*/
fun playRedstone(player: Player, location: Location, color: Color) {
playColoured(REDSTONE, player, location, color)
}
/**
* @see playColoured
*/
fun playRedstone(location: Location, color: Color) {
playColoured(REDSTONE, location, color)
}
/**
* @see playColoured
*/
inline fun playRedstone(
location: Location,
color: Color,
predicate: (Player) -> Boolean
) = playColoured(REDSTONE, location, color, predicate)
/**
* @see playColoured
*/
fun playMobSpell(player: Player, location: Location, color: Color, ambient: Boolean = false) {
playColoured(if (ambient) SPELL_MOB_AMBIENT else SPELL_MOB, player, location, color)
}
/**
* @see playColoured
*/
fun playMobSpell(location: Location, color: Color, ambient: Boolean = false) {
playColoured(if (ambient) SPELL_MOB_AMBIENT else SPELL_MOB, location, color)
}
/**
* @see playColoured
*/
inline fun playMobSpell(
location: Location,
color: Color,
ambient: Boolean = false,
predicate: (Player) -> Boolean
) = playColoured(if (ambient) SPELL_MOB_AMBIENT else SPELL_MOB, location, color, predicate)
/**
* Plays a [ITEM_CRACK] particle type with the specified [material] in the given location
* for only to the specified [player].
*
* @param player the unique player that's will be able to see the particle.
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
fun playItemBreak(
player: Player,
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
) = ITEM_CRACK.play(
player,
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF)
)
/**
* Plays a [ITEM_CRACK] particle type with the specified [material] in the given location
* for all players in the location world.
*
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
fun playItemBreak(
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
) = ITEM_CRACK.play(
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF)
)
/**
* Plays a [ITEM_CRACK] particle type with the specified [material] in the given location
* for all players in the location world that's satisfies the [predicate].
*
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
inline fun playItemBreak(
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
predicate: (Player) -> Boolean
) = ITEM_CRACK.play(
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF),
predicate = predicate
)
/**
* Plays a [BLOCK_CRACK] particle type with the specified [material] in the given location
* for only to the specified [player].
*
* @param player the unique player that's will be able to see the particle.
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
fun playBlockBreak(
player: Player,
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
) = BLOCK_CRACK.play(
player,
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF)
)
/**
* Plays a [BLOCK_CRACK] particle type with the specified [material] in the given location
* for all players in the location world.
*
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
fun playBlockBreak(
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
) = BLOCK_CRACK.play(
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF)
)
/**
* Plays a [BLOCK_CRACK] particle type with the specified [material] in the given location
* for all players in the location world that's satisfies the [predicate].
*
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
inline fun playBlockBreak(
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
predicate: (Player) -> Boolean
) = BLOCK_CRACK.play(
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF),
predicate = predicate
)
/**
* Plays a [BLOCK_DUST] particle type with the specified [material] in the given location
* for only to the specified [player].
*
* @param player the unique player that's will be able to see the particle.
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
fun playBlockDust(
player: Player,
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
) = BLOCK_DUST.play(
player,
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF)
)
/**
* Plays a [BLOCK_DUST] particle type with the specified [material] in the given location
* for all players in the location world.
*
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
fun playBlockDust(
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
) = BLOCK_DUST.play(
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF)
)
/**
* Plays a [BLOCK_DUST] particle type with the specified [material] in the given location
* for all players in the location world that's satisfies the [predicate].
*
* @param material the material that's the particle will be shown.
* @param location the location that the particle will spawn.
*/
inline fun playBlockDust(
location: Location,
material: Materials,
amount: Int = 1,
speed: Float = 0f,
offsetX: Float = 0f,
offsetY: Float = 0f,
offsetZ: Float = 0f,
predicate: (Player) -> Boolean
) = BLOCK_DUST.play(
location,
amount,
speed,
offsetX,
offsetY,
offsetZ,
material.subdata shl 12 or (material.id and 0xFFF),
predicate = predicate
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy