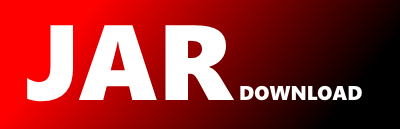
walkmc.Plugin.kt Maven / Gradle / Ivy
package walkmc
import kotlinx.coroutines.*
import kotlinx.serialization.*
import org.bukkit.entity.*
import org.bukkit.event.*
import org.bukkit.plugin.java.*
import org.bukkit.plugin.messaging.*
import walkmc.annotation.*
import walkmc.serializer.*
import kotlin.coroutines.*
import java.util.*
/**
* Represents an extendable [JavaPlugin].
*
* [Plugin] have built-in support for:
* * [CoroutineScope]
* * [Listener]
* * [PluginMessageListener]
* * [ResourceLoader]
*/
abstract class Plugin : JavaPlugin(), CoroutineScope, Listener, PluginMessageListener, ResourceLoader {
override val coroutineContext: CoroutineContext = EmptyCoroutineContext
/**
* All registered resources from this loader.
*/
override val resources: MutableList> = LinkedList()
/**
* Execution when this plugin is loading.
*/
open fun load() = Unit
/**
* Execution when this plugin starts.
*/
open fun start() = Unit
/**
* Execution when this plugin disables.
*/
open fun disable() = Unit
override fun onLoad() {
updateResources()
load()
triggerResource(Load.LOAD)
}
override fun onEnable() {
start()
triggerResource(Load.ENABLE)
}
override fun onDisable() {
disable()
triggerResource(Load.DISABLE)
runCatching {
cancel()
}
}
/**
* Gets a raw list of all registered resources processed by the resource processor.
*/
override fun getRawResources(): List {
val raw = getResource("resources.json")?.reader()?.buffered().use { it?.readText() }
return if (raw.isNullOrBlank()) emptyList() else Json.decodeFromString(raw)
}
override fun onPluginMessageReceived(channel: String, player: Player, message: ByteArray) = Unit
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy