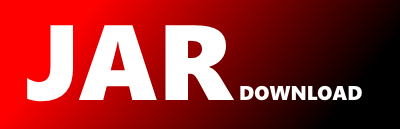
walkmc.Slot.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc
import kotlinx.serialization.*
import org.bukkit.*
import org.bukkit.entity.*
import org.bukkit.inventory.*
import org.bukkit.inventory.meta.*
import org.bukkit.material.*
import walkmc.extensions.*
import walkmc.placeholder.*
/**
* A Slot represents a storable index and item for uses with inventory.
* This contains the index of the slot and the item of the slot.
*/
@Serializable
data class Slot(var slot: Int, var item: @Contextual ItemStack)
/**
* Gives this slot representation to the specified inventory.
*/
inline fun Slot.give(inventory: Inventory) = inventory.setItem(slot, item)
/**
* Gives this slot representation to the specified player.
*/
inline fun Slot.give(player: Player) = player.inventory.setItem(slot, item)
/**
* Copies this slot and applies the [block] function to this.
*/
inline fun Slot.copy(block: Slot.() -> Unit) = copy().apply(block)
/**
* Creates a [Slot] by this item and the specified [slot].
*/
inline infix fun ItemStack.slot(slot: Int) = Slot(slot, this)
/**
* Changes this item stack material type by the specified [material].
*/
fun Slot.changeToMaterial(material: Materials): Slot {
item.material = material
return this
}
/**
* Changes this item stack to the specified head representation of [url].
*/
fun Slot.changeToHead(url: String): Slot {
item.material = Materials.PLAYER_SKULL
item.data = SkullItem(url)
item.applyMetaTo {
this.url = url
}
return this
}
/**
* Changes this item stack to the specified head representation of the owner [name].
*/
fun Slot.changeToHeadOwner(name: String): Slot {
item.material = Materials.PLAYER_SKULL
item.applyMetaTo {
this.owner = name
}
return this
}
/**
* Changes this item stack to the specified head representation of the owner [player].
*/
fun Slot.changeToHeadOwner(player: OfflinePlayer): Slot {
item.material = Materials.PLAYER_SKULL
item.applyMetaTo {
this.owner = player.name
}
return this
}
/**
* Copies and changes this item stack material type by the specified [material].
*/
fun Slot.copyToMaterial(material: Materials) = copy {
item.material = material
}
/**
* Copies and changes this item stack to the specified head representation of [url].
*/
fun Slot.copyToHead(url: String) = copy {
item.material = Materials.PLAYER_SKULL
item.data = SkullItem(url)
item.applyMetaTo {
this.url = url
}
}
/**
* Copies and changes this item stack to the specified head representation of the owner [name].
*/
fun Slot.copyToHeadOwner(name: String) = copy {
item.material = Materials.PLAYER_SKULL
item.applyMetaTo {
owner = name
}
}
/**
* Copies and changes this item stack to the specified head representation of the owner [player].
*/
fun Slot.copyToHeadOwner(player: OfflinePlayer) = copy {
item.material = Materials.PLAYER_SKULL
item.applyMetaTo {
owner = player.name
}
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun Slot.process(model: ItemStack, placeholder: Placeholder, value: T) = apply {
item.process(model, placeholder, value)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun Slot.process(model: ItemStack, value: Pair) = apply {
item.process(model, value)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun Slot.process(model: ItemStack, vararg value: Pair) = apply {
item.process(model, *value)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore.
*/
fun Slot.process(model: ItemStack, value: Map) = apply {
item.process(model, value)
}
/**
* Process this item stack with the specified placeholder and value.
*/
fun Slot.process(placeholder: Placeholder, value: T) = apply {
item.process(placeholder, value)
}
/**
* Process only specified placeholder in this item stack.
*/
fun Slot.process(value: Pair) = apply {
item.process(value)
}
/**
* Process all specifieds placeholders in this item stack.
*/
fun Slot.process(vararg values: Pair) = apply {
item.process(*values)
}
/**
* Process all specifieds placeholders in this item stack.
*/
fun Slot.process(values: Map) = apply {
item.process(values)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun Slot.processCopy(model: ItemStack, placeholder: Placeholder, value: T) = copy {
item.process(model, placeholder, value)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun Slot.processCopy(model: ItemStack, value: Pair) = copy {
item.process(model, value)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun Slot.processCopy(model: ItemStack, vararg value: Pair) = copy {
item.process(model, *value)
}
/**
* Process this item stack with the specified placeholder and value
* by the model name and lore and returns a copy of them.
*/
fun Slot.processCopy(model: ItemStack, value: Map) = copy {
item.process(model, value)
}
/**
* Process this item stack with the specified placeholder and value and returns a copy of them.
*/
fun Slot.processCopy(placeholder: Placeholder, value: T) = copy {
item.process(placeholder, value)
}
/**
* Process only specified placeholder in this item stack and returns a copy of them.
*/
fun Slot.processCopy(value: Pair) = copy {
item.process(value)
}
/**
* Process all specifieds placeholders in this item stack and returns a copy of them.
*/
fun Slot.processCopy(vararg values: Pair) = copy {
item.process(*values)
}
/**
* Process all specifieds placeholders in this item stack and returns a copy of them.
*/
fun Slot.processCopy(values: Map) = copy {
item.process(values)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy