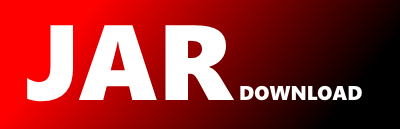
walkmc.Task.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package walkmc
import org.bukkit.scheduler.*
import walkmc.extensions.*
/**
* Represents a scheduled task.
*/
open class Task(var action: Task.() -> Unit) : BukkitRunnable() {
/**
* Returns if this task is running.
*/
var isRunning: Boolean = false
private set
/**
* Returns if this task is cancelled.
*/
var isCancelled: Boolean = false
private set
/**
* The execution of this task.
*/
override fun run() {
isRunning = true
action()
}
/**
* Cancels this task.
*/
override fun cancel() {
if (!isRunning)
return
isRunning = false
isCancelled = true
super.cancel()
}
}
/**
* Represents a scheduled task that's only executes certain amount of times.
*/
open class CountTask(
var maxCount: Int,
action: CountTask.() -> Unit
) : Task(action.cast()) {
/**
* The current count of this task.
*/
var count = 0
/**
* The execution of this task.
*/
override fun run() {
if (count >= maxCount)
cancel()
count++
super.run()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy