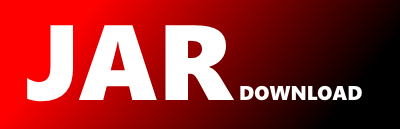
walkmc.cache.ExpirableMap.kt Maven / Gradle / Ivy
package walkmc.cache
import net.jodah.expiringmap.*
import walkmc.extensions.*
import kotlin.time.*
import java.util.concurrent.*
/**
* A [ExpiringMap] cache implementation.
*/
open class ExpirableMap(
delegate: ExpiringMap = ExpiringMap.builder().variableExpiration().build(),
) : Cache(delegate) {
val expirableDelegate = delegate
/**
* Submits a key-value in this expiring registry with the
* specified expiring duration.
*/
fun put(
key: K,
value: V,
duration: Long,
unit: TimeUnit,
policy: ExpirationPolicy = ExpirationPolicy.CREATED,
): V = expirableDelegate.put(key, value, policy, duration, unit)
/**
* Submits a key-value in this expiring registry with the
* specified expiring duration.
*/
fun put(key: K, value: V, duration: Duration, policy: ExpirationPolicy = ExpirationPolicy.CREATED): V =
expirableDelegate.put(key, value, policy, duration.inWholeMilliseconds, TimeUnit.MILLISECONDS)
/**
* Resets the expiration time of a key.
*/
fun reset(key: K) = expirableDelegate.resetExpiration(key)
/**
* Gets the expirition time of this key.
*/
fun getExpirataion(key: K): Long = expirableDelegate.getExpiration(key)
/**
* Gets the expected expirition time of this key.
*/
fun expiresIn(key: K): Duration = milliseconds(expirableDelegate.getExpectedExpiration(key))
/**
* Adds a listener to be triggered when a key expires.
* This runs synchronously.
*/
fun onExpire(isAsync: Boolean = false, action: (K, V) -> Unit) {
if (isAsync)
expirableDelegate.addAsyncExpirationListener(action)
else
expirableDelegate.addExpirationListener(action)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy