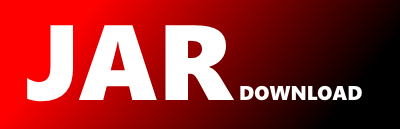
walkmc.collections.LimitedSet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
package walkmc.collections
import java.util.*
/**
* Represents a limited set. A limited set only can have specified [limit] of elements.
*/
open class LimitedSet(
var limit: Int,
private val delegate: MutableSet = HashSet()
) : MutableSet by delegate {
/**
* Adds the specified element to the set.
*
* @return false if the size of this set is reached to [limit]
* or the element is already added, otherwise, true
*/
override fun add(element: T): Boolean {
if (size >= limit)
return false
return delegate.add(element)
}
/**
* Adds all of the elements of the specified collection to this collection.
*
* The elements are appended in the order they appear in the elements collection.
*/
override fun addAll(elements: Collection): Boolean {
if (size >= limit)
return false
return delegate.addAll(elements.take(limit - size))
}
}
/**
* Represents a limited tree set. A limited tree set only can have specified [limit] of elements.
*/
open class LimitedTreeSet(
var limit: Int,
comparator: Comparator? = null
) : TreeSet(comparator) {
constructor(
limit: Int,
from: Collection,
comparator: Comparator? = null
) : this(limit, comparator) { addAll(from) }
/**
* Adds the specified element to the set.
*
* @return false if the size of this set is reached to [limit]
* or the element is already added, otherwise, true
*/
override fun add(element: T): Boolean {
if (size >= limit)
return false
return super.add(element)
}
/**
* Adds all of the elements of the specified collection to this collection.
*
* The elements are appended in the order they appear in the elements collection.
*/
override fun addAll(elements: Collection): Boolean {
if (size >= limit)
return false
return if (comparator() == null) {
super.addAll(elements.sortedWith(compareBy { it as Comparable<*> }).take(limit - size))
} else {
super.addAll(elements.sortedWith(comparator()).take(limit - size))
}
}
}
/**
* Creates a empty limited tree set.
*/
fun > limitedTreeSetOf(limit: Int) = LimitedTreeSet(limit)
/**
* Creates a limited tree set by [elements].
*/
fun > limitedTreeSetOf(limit: Int, vararg elements: T) =
LimitedTreeSet(limit, elements.toMutableSet().sorted())
/**
* Creates a empty limited tree set.
*/
fun limitedTreeSetOf(limit: Int, comparator: Comparator) = LimitedTreeSet(limit, comparator)
/**
* Creates a limited tree set by [elements] with the given [comparator].
*/
fun limitedTreeSetOf(limit: Int, comparator: Comparator, vararg elements: T) =
LimitedTreeSet(limit, elements.toMutableSet().sortedWith(comparator), comparator)
/**
* Converts this iterable to a limited set.
*/
fun Iterable.toLimitedSet(limit: Int) = LimitedSet(limit, take(limit).toMutableSet())
/**
* Converts this iterable to a limited tree set.
*/
fun Iterable.toLimitedTreeSet(
limit: Int,
comparator: Comparator
) = LimitedTreeSet(limit, take(limit), comparator)
/**
* Converts this iterable to a limited tree set.
*/
fun > Iterable.toLimitedTreeSet(limit: Int) = LimitedTreeSet(limit, take(limit))
© 2015 - 2025 Weber Informatics LLC | Privacy Policy