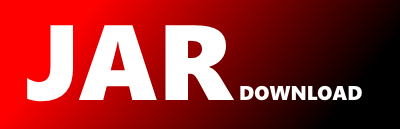
walkmc.extensions.Blocks.kt Maven / Gradle / Ivy
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions
import org.bukkit.*
import org.bukkit.block.*
import org.bukkit.material.*
import walkmc.serializer.tag.impl.*
/**
* Gets the material data represented by this block.
*/
inline var Block.materialData: MaterialData
get() = newData(type, data)
set(value) {
type = value.itemType
data = value.data
}
/**
* Sets the new material data of this block by builder style
*/
inline fun Block.materialData(data: MaterialData) = apply {
materialData = data
}
/**
* Sets the new material data of this block by builder style
*/
inline fun Block.materialData(type: Material, data: Number) = apply {
materialData = newData(type, data)
}
/**
* Sets the new data of this block by builder style
*/
inline fun Block.data(data: Number) = apply {
this.data = data.toByte()
}
/**
* Sets the new type of this block by builder style
*/
inline fun Block.type(type: Material) = apply {
this.type = type
}
/**
* Gets the up relative block of this block.
*/
inline fun Block.up(distance: Int = 1): Block = getRelative(0, distance, 0)
/**
* Gets the down relative block of this block.
*/
inline fun Block.down(distance: Int = 1): Block = getRelative(0, -distance, 0)
/**
* Gets the south relative block of this block.
*/
inline fun Block.south(distance: Int = 1): Block = getRelative(BlockFace.SOUTH, distance)
/**
* Gets the north relative block of this block.
*/
inline fun Block.north(distance: Int = 1): Block = getRelative(BlockFace.NORTH, distance)
/**
* Gets the east relative location of this location.
*/
inline fun Block.east(distance: Int = 1): Block = getRelative(BlockFace.EAST, distance)
/**
* Gets the west relative block of this block.
*/
inline fun Block.west(distance: Int = 1): Block = getRelative(BlockFace.WEST, distance)
/**
* Gets the south west relative block of this block.
*/
inline fun Block.southwest(distance: Int = 1): Block = getRelative(BlockFace.SOUTH_WEST, distance)
/**
* Gets the north west relative block of this block.
*/
inline fun Block.northwest(distance: Int = 1): Block = getRelative(BlockFace.NORTH_WEST, distance)
/**
* Gets the south east relative block of this block.
*/
inline fun Block.southeast(distance: Int = 1): Block = getRelative(BlockFace.SOUTH_EAST, distance)
/**
* Gets the north east relative block of this block.
*/
inline fun Block.northeast(distance: Int = 1): Block = getRelative(BlockFace.NORTH_EAST, distance)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy