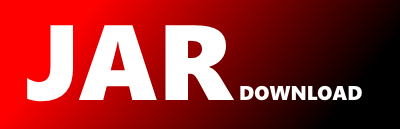
walkmc.extensions.Entities.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions
import net.minecraft.server.*
import org.bukkit.craftbukkit.entity.*
import org.bukkit.entity.*
import org.bukkit.entity.Entity
import org.bukkit.util.*
import walkmc.*
import walkmc.nbt.*
import walkmc.serializer.tag.impl.*
/**
* Get the craft handler of this entity.
*/
inline val Entity.craftHandler get() = this as CraftEntity
/**
* Get the minecraft handler of this entity.
*/
inline val Entity.handler: MinecraftEntity get() = craftHandler.handle
/**
* Gets the bounding box of this entity.
*/
var Entity.boundingBox: BoundingBox
get() = BoundingBox(axis)
set(value) {
handler.boundingBox = value.toAxis()
}
/**
* Gets the [AxisAlignedBB] of this entity.
*/
var Entity.axis: AxisAlignedBB
get() = handler.boundingBox
set(value) {
handler.boundingBox = value
}
/**
* Applies the given [block] to the storage tag of this entity.
*/
inline fun Entity.applyStorage(block: CompoundTag.() -> Unit) = apply {
val handler = handler
val tag = handler.customTag.apply(block)
handler.customTag = tag
}
/**
* Gets the tag storage of this entity.
*
* ## NOTE:
*
* This MUST be used in almost all cases when configuring persistent data or custom data of this entity.
* Certain things like, setting this item to [isPersistent] must be configured with [tag].
*
* For convenience, prefers using this almost in all cases.
*/
var Entity.storage: CompoundTag
get() = handler.customTag
set(value) {
val handler = handler
handler.customTag = value
}
/**
* Applies and changes the velocity of this player by the specified [velo].
*/
inline fun Entity.applyVelocity(velo: Vector = velocity, block: Vector.() -> Unit) {
velocity = velo.apply(block)
}
/**
* Gets the entity root tag.
*
* ## NOTE:
*
* This MUST only used to configure certains aspects of persistent data or item data that's
* is not available in [storage], like [isPersistent] and others.
*
* For convenience, prefers using [storage] almost in all cases.
*/
inline val Entity.tag: NBTGroup
get() = EntityTag(this)
/**
* Transforms this entity root tag by the specified transform.
*/
inline fun Entity.applyTag(transform: NBTTagCompound.() -> Unit) =
handler.f(tag.apply(transform))
/**
* Returns if this entity is silent or not.
*/
var Entity.isSilent: Boolean
get() = tag.getBoolean("Silent")
set(value) {
applyTag {
setBoolean("Silent", value)
}
}
/**
* Returns if this entity is persistent or not.
*/
var Entity.isPersistent: Boolean
get() = tag.getBoolean("PersistenceRequired")
set(value) {
applyTag {
setBoolean("PersistenceRequired", value)
}
}
/**
* Returns if this entity is invulnerable or not.
*/
var Entity.isInvulnerable: Boolean
get() = tag.getBoolean("Invulnerable")
set(value) {
applyTag {
setBoolean("Invulnerable", value)
}
}
/**
* Returns if this entity has artificial intelligence or not.
*/
var Entity.hasAI: Boolean
get() = !tag.getBoolean("NoAI")
set(value) {
applyTag {
setBoolean("NoAI", !value)
}
}
/**
* Returns if this entity is baby or not.
*/
var Entity.isBaby: Boolean
get() = when (this) {
is Ageable -> !isAdult
is Zombie -> isBaby
else -> false
}
set(value) = when (this) {
is Ageable -> if (value) setBaby() else setAdult()
is Zombie -> isBaby = value
else -> Unit
}
/**
* Returns if this entity is adult or not.
*/
var Entity.isAdult: Boolean
get() = !isBaby
set(value) {
isBaby = !value
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy