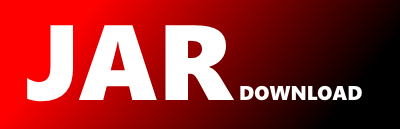
walkmc.extensions.Events.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions
import kotlinx.coroutines.*
import org.bukkit.event.*
import org.bukkit.event.block.*
import org.bukkit.event.player.*
import org.bukkit.plugin.Plugin
import walkmc.*
import kotlin.reflect.*
/**
* Calls this event.
*/
inline fun T.call(): T {
pluginManager.callEvent(this)
return this
}
/**
* Returns if the action of this interact event is any type of left click.
*/
val PlayerInteractEvent.isLeftClick: Boolean
get() = action == Action.LEFT_CLICK_AIR || action == Action.LEFT_CLICK_BLOCK
/**
* Returns if the action of this interact event is any type of right click.
*/
val PlayerInteractEvent.isRightClick: Boolean
get() = action == Action.RIGHT_CLICK_AIR || action == Action.RIGHT_CLICK_BLOCK
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Listener.listening(
type: KClass,
plugin: Plugin,
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
crossinline action: T.() -> Unit,
) = pluginManager.registerEvent(type.java, this, priority, { _, event ->
if (type.isInstance(event))
action(event.cast())
}, plugin, ignoreCancelled)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Listener.listening(
plugin: Plugin,
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
noinline action: T.() -> Unit,
) = listening(T::class, plugin, priority, ignoreCancelled, action)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Plugin.listening(
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
noinline action: T.() -> Unit,
) = StandardListener().listening(this, priority, ignoreCancelled, action)
/**
* Register a event listener with the specified event in generic type in this server.
*/
fun Listener.subscribe(
type: KClass,
plugin: Plugin,
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
action: T.() -> Unit,
) = pluginManager.registerEvent(type.java, this, priority, { _, event ->
if (type.isInstance(event))
action(event.cast())
}, plugin, ignoreCancelled)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Listener.subscribe(
plugin: Plugin,
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
noinline action: T.() -> Unit,
) = subscribe(T::class, plugin, priority, ignoreCancelled, action)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Plugin.subscribe(
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
noinline action: T.() -> Unit,
) = StandardListener().subscribe(this, priority, ignoreCancelled, action)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Listener.subscribeSuspend(
type: KClass,
plugin: walkmc.Plugin,
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
crossinline action: suspend T.() -> Unit,
) = pluginManager.registerEvent(type.java, this, priority, { _, event ->
if (type.isInstance(event)) {
plugin.launch {
action(event.cast())
}
}
}, plugin, ignoreCancelled
)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun Listener.subscribeSuspend(
plugin: walkmc.Plugin,
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
noinline action: suspend T.() -> Unit,
) = subscribeSuspend(T::class, plugin, priority, ignoreCancelled, action)
/**
* Register a event listener with the specified event in generic type in this server.
*/
inline fun walkmc.Plugin.subscribeSuspend(
priority: EventPriority = EventPriority.NORMAL,
ignoreCancelled: Boolean = false,
noinline action: suspend T.() -> Unit,
) = StandardListener().subscribeSuspend(this, priority, ignoreCancelled, action)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy