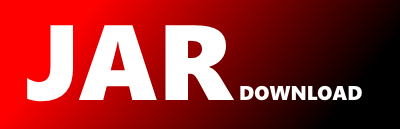
walkmc.extensions.Players.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions
import com.mojang.authlib.*
import net.md_5.bungee.api.chat.*
import net.minecraft.server.*
import org.bukkit.*
import org.bukkit.Material
import org.bukkit.block.Block
import org.bukkit.craftbukkit.entity.*
import org.bukkit.entity.*
import org.bukkit.inventory.ItemStack
import org.bukkit.util.*
import walkmc.*
import walkmc.extensions.constants.*
import walkmc.serializer.tag.impl.*
import walkmc.time.*
import kotlin.time.*
import java.util.*
/**
* Represents all possible staff level groups.
*/
enum class StaffLevel(val permission: String, val level: Int) {
MEMBER("", 0),
HELPER("walk.staff.helper", 10),
MODERATOR("walk.staff.mod", 20),
COORDENATOR("walk.staff.coord", 30),
MANAGER("walk.staff.manager", 40),
ADMIN("walk.staff.admin", 50);
companion object {
operator fun get(player: Player) = when {
player.isOp || player.hasPermission(ADMIN.permission) -> ADMIN
player.hasPermission(MANAGER.permission) -> MANAGER
player.hasPermission(COORDENATOR.permission) -> COORDENATOR
player.hasPermission(MODERATOR.permission) -> MODERATOR
player.hasPermission(HELPER.permission) -> HELPER
else -> MEMBER
}
}
}
/**
* Represents all possible vip level groups.
*/
enum class VipLevel(val permission: String, val level: Int) {
MEMBER("", 0),
BARON("vip.baron", 10),
DUKE("vip.duke", 20),
PRINCE("vip.prince", 30),
WALK("vip.walk", 40);
companion object {
operator fun get(player: Player) = when {
player.isOp || player.isManager || player.hasPermission(WALK.permission) -> WALK
player.hasPermission(PRINCE.permission) -> PRINCE
player.hasPermission(DUKE.permission) -> DUKE
player.hasPermission(BARON.permission) -> BARON
else -> MEMBER
}
}
}
/**
* Gets the staff level of this player.
*/
inline val Player.staffLevel: StaffLevel
get() = StaffLevel[this]
/**
* Gets the vip level of this player.
*/
inline val Player.vipLevel: VipLevel
get() = VipLevel[this]
/**
* Verifies if the staff level of this player is ADMIN
*/
val Player.isAdmin: Boolean
get() = staffLevel.level >= StaffLevel.ADMIN.level
/**
* Verifies if the staff level of this player is MANAGER
*/
val Player.isManager: Boolean
get() = staffLevel.level >= StaffLevel.MANAGER.level
/**
* Verifies if the staff level of this player is COORDENATOR
*/
val Player.isCoordenator: Boolean
get() = staffLevel.level >= StaffLevel.COORDENATOR.level
/**
* Verifies if the staff level of this player is MODERATOR
*/
val Player.isModerator: Boolean
get() = staffLevel.level >= StaffLevel.MODERATOR.level
/**
* Verifies if the staff level of this player is HELPER
*/
val Player.isHelper: Boolean
get() = staffLevel.level >= StaffLevel.HELPER.level
/**
* Verifies if the vip level of this player is of the vip WALK.
*/
val Player.isWalk: Boolean
get() = vipLevel.level >= VipLevel.WALK.level
/**
* Verifies if the vip level of this player is of the vip PRINCE.
*/
val Player.isPrince: Boolean
get() = vipLevel.level >= VipLevel.PRINCE.level
/**
* Verifies if the vip level of this player is of the vip DUKE.
*/
val Player.isDuke: Boolean
get() = vipLevel.level >= VipLevel.DUKE.level
/**
* Verifies if the vip level of this player is of the vip BARON.
*/
val Player.isBaron: Boolean
get() = vipLevel.level >= VipLevel.BARON.level
/**
* Returns the game profile of this player.
*/
val Player.profile: GameProfile
get() = handler.profile
/**
* Gets the local storage of this player. Used to declare permanent data.
*/
val OfflinePlayer.localStorage: CompoundTag
get() = uuid.playerLocalStorage
/**
* Gets the local storage of the player represented by this uuid. Used to declare permanent data.
*/
val UUID.playerLocalStorage: CompoundTag
get() = PlayerLocalStorage.getOrPut(this) { CompoundTag() }
/**
* Gets the local storage of this player. Used to declare temporary data.
*/
val OfflinePlayer.sessionStorage: Storage
get() = uuid.playerSessionStorage
/**
* Gets the local storage of the player represented by this uuid. Used to declare temporary data.
*/
val UUID.playerSessionStorage: Storage
get() = PlayerSessionStorage.getOrPut(this) { Storage() }
/**
* Saves the tag of this player.
*/
fun OfflinePlayer.savePlayerStorage() = uuid.savePlayerStorage()
/**
* Loads the tag of this player.
*/
fun OfflinePlayer.loadPlayerStorage() = uuid.loadPlayerStorage()
/**
* Saves the tag of this player.
*/
fun UUID.savePlayerStorage() = PlayerLocalStorage.save(this)
/**
* Loads the tag of this player.
*/
fun UUID.loadPlayerStorage() = PlayerLocalStorage.load(this)
/**
* Gets the craft handler of this player.
*/
inline val Player.craftHandler get() = this as CraftPlayer
/**
* Gets the minecraft handler of this player.
*/
inline val Player.handler: EntityPlayer get() = craftHandler.handle
/**
* Shortcut for getting the UUID of this player.
*/
inline val OfflinePlayer.uuid: UUID get() = uniqueId
/**
* Returns the ip of this player.
*/
inline val Player.ip: String get() = address.hostName
/**
* Returns the head of this player.
*/
inline val OfflinePlayer.head: ItemStack get() = newHeadOwner(name)
/**
* Gets the repository file of this player.
*/
val OfflinePlayer.repository get() = newFile(RepositoryFolder, "$uuid.dat")
/**
* Makes the player says a message in the chat.
*/
inline fun Player.say(message: String) = chat(message)
/**
* Gives a item to the player inventory.
*/
inline fun Player.giveItem(item: ItemStack): Map = inventory.addItem(item)
/**
* Clears completely the inventory of this inventory, including the armor content.
*/
fun Player.clearInventory() {
inventory.armorContents = null
inventory.clear()
}
/**
* Gets the current held slot of this player.
*/
inline var Player.heldSlot: Int
get() = inventory.heldItemSlot
set(value) {
inventory.heldItemSlot = value
}
/**
* Sends a packet to this player.
*/
fun Player.sendPacket(packet: Packet) = handler.playerConnection.sendPacket(packet)
/**
* Sends a action bar to this player.
*/
fun Player.action(message: Any) {
sendPacket(PacketPlayOutChat(createChatComponent(message.toString()), 2.toByte()))
}
/**
* Sends a title to this player.
*/
fun Player.title(
title: String = "",
subtitle: String = "",
fadeIn: Int = 20,
stay: Int = 40,
fadeOut: Int = 20,
) {
sendPacket(PacketPlayOutTitle(TITLE, createChatComponent(title)))
sendPacket(PacketPlayOutTitle(SUBTITLE, createChatComponent(subtitle)))
sendPacket(PacketPlayOutTitle(fadeIn, stay, fadeOut))
}
/**
* Sends a tablist update packet to this player.
*/
fun Player.tablist(
header: String,
footer: String
) = sendPacket(PacketPlayOutPlayerListHeaderFooter(createChatComponent(header), createChatComponent(footer)))
/**
* Plays a sound for this player.
*/
fun Player.playSound(sound: Sound, volume: Float, pitch: Float) {
playSound(location, sound, volume, pitch)
}
/**
* Logs a notification to this player.
*/
inline fun Player.log(notification: Notification) = notification.log(this)
/**
* Logs a text component to this player.
*/
inline fun Player.log(component: TextComponent) = spigot().sendMessage(component)
/**
* Gets the target block of this player. This not classifies AIR blocks as target.
* @see getTargetBlockAir
*/
fun Player.getTargetBlock(range: Int): Block? {
val iter = BlockIterator(this, range)
var lastBlock = iter.next()
while (iter.hasNext()) {
lastBlock = iter.next()
if (lastBlock.type === Material.AIR) continue
break
}
return lastBlock
}
/**
* Gets the target block of this player. This classifies AIR blocks as target.
*/
fun Player.getTargetBlockAir(range: Int): Block {
val iterator = BlockIterator(this, range)
var current = 0
var lastBlock = iterator.next()
while (current++ < range || iterator.hasNext())
lastBlock = iterator.next()
return lastBlock
}
/**
* Gets the target location of this player. This classifies AIR blocks as target.
*/
fun Player.getTargetLocation(range: Int) = getTargetBlockAir(range).location
/**
* Submits a new cooldown to this player with the specified key and duration.
*/
fun Player.addDelay(key: String, duration: Duration) = DelayManager.put(this, key, duration)
/**
* Locates a cooldown with the specified key of this player.
*/
fun Player.getDelay(key: String): Delay = DelayManager.locate(this, key) ?: EmptyDelay
/**
* Gets the duration of a delay with the specified key of this player.
*/
fun Player.delayDuration(key: String): Long = getDelay(key).expiresIn
/**
* Resets the delay duration of the specified key of this player.
*/
fun Player.redefineDelay(key: String) {
getDelay(key).redefine()
}
/**
* Verifies if the cooldown of this player with the specified key is expired.
*/
fun Player.isDelayExpired(key: String): Boolean = getDelay(key).isExpired
/**
* Removes a cooldown of a player by the specified key.
*/
fun Player.removeDelay(key: String) = DelayManager.remove(this, key)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy