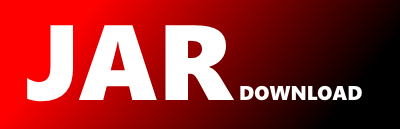
walkmc.extensions.Plugins.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions
import kotlinx.coroutines.*
import org.bukkit.plugin.Plugin
import walkmc.*
import kotlin.time.*
/**
* Logs a message to console of this server by this plugin.
*/
fun Plugin.log(message: Any) = console.log("§7[§e${name}§7] §f$message")
/**
* Logs an error message to console of this server by this plugin.
*/
fun Plugin.logError(message: Any) = console.log("§7[§e${name}§7] §cERROR §7- §f$message")
/**
* Logs a warning message to console of this server by this plugin.
*/
fun Plugin.logWarning(message: Any) = console.log("§7[§e${name}§7] §eWARNING §7- §f$message")
/**
* Schedules a task with this plugin.
*/
fun Plugin.schedule(async: Boolean = false, action: Task.() -> Unit): Task {
val task = Task(action)
if (async) task.runTaskAsynchronously(this) else task.runTask(this)
return task
}
/**
* Schedules a later task with this plugin.
*/
fun Plugin.scheduleLater(
delay: Long,
async: Boolean = false,
action: Task.() -> Unit
): Task {
val task = Task(action)
if (async)
task.runTaskLaterAsynchronously(this, delay / 50)
else
task.runTaskLater(this, delay / 50)
return task
}
/**
* Schedules a later task with this plugin.
*/
fun Plugin.scheduleLater(
delay: Duration,
async: Boolean = false,
action: Task.() -> Unit
): Task = scheduleLater(delay.inWholeMilliseconds, async, action)
/**
* Schedules a timer task with this plugin.
*/
fun Plugin.scheduleTimer(
period: Long,
delay: Long = 0,
async: Boolean = false,
action: Task.() -> Unit
): Task {
val task = Task(action)
if (async)
task.runTaskTimerAsynchronously(this, delay / 50, period / 50)
else
task.runTaskTimer(this, delay / 50, period / 50)
return task
}
/**
* Schedules a timer task with this plugin.
*/
fun Plugin.scheduleTimer(
period: Duration,
delay: Duration = milliseconds(0),
async: Boolean = false,
action: Task.() -> Unit
): Task = scheduleTimer(period.inWholeMilliseconds, delay.inWholeMilliseconds, async, action)
/**
* Schedules a timer count task with this plugin.
*/
fun Plugin.scheduleTimer(
period: Long,
count: Int,
delay: Long = 0,
async: Boolean = false,
action: CountTask.() -> Unit
): CountTask {
val task = CountTask(count, action)
if (async)
task.runTaskTimerAsynchronously(this, delay / 50, period / 50)
else
task.runTaskTimer(this, delay / 50, period / 50)
return task
}
/**
* Schedules a timer count task with this plugin.
*/
fun Plugin.scheduleTimer(
period: Duration,
count: Int,
delay: Duration = milliseconds(0),
async: Boolean = false,
action: CountTask.() -> Unit
): CountTask =
scheduleTimer(period.inWholeMilliseconds, count, delay.inWholeMilliseconds, async, action)
/**
* Launchs a new coroutine that's will be executed every [delay] while [doWhile] is true.
*
* @param delay the duration between every next execution
* @param doWhile if true, the execution will be performed, if false, will be ended.
*/
inline fun CoroutineScope.launch(
delay: Duration,
doWhile: Boolean = true,
crossinline block: suspend CoroutineScope.() -> Unit
) = launch {
while (doWhile) {
block(this)
delay(delay)
}
}
/**
* Launchs a new coroutine that's will be executed for [amount] times every [delay].
*
* @param delay the duration between every next execution
* @param amount the amount of times to repeat
*/
inline fun CoroutineScope.launch(
delay: Duration,
amount: Int,
crossinline block: suspend CoroutineScope.(Int) -> Unit
) = launch {
repeat(amount) {
block(this, it)
delay(delay)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy