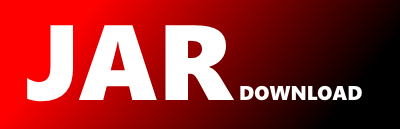
walkmc.extensions.Times.kt Maven / Gradle / Ivy
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions
import com.soywiz.klock.*
import walkmc.extensions.numbers.*
import kotlin.time.Duration
import java.text.*
import java.text.DateFormat
import java.time.*
import java.util.Date
/**
* The default date format used.
*/
val DEFAULT_DATE_FORMAT = SimpleDateFormat("HH:mm:ss dd/MM/yyyy")
/**
* Returns the current time millis.
*/
inline val currentMillis get() = System.currentTimeMillis()
/**
* Gets the actual date, this is, the current date.
*/
inline fun now(): DateTime = DateTime.now()
/**
* Converts this date to a date time.
*/
inline fun Date.toDateTime(): DateTime = DateTime(time)
/**
* Converts this date to a local date.
*/
inline fun Date.toLocalDateTime(): LocalDateTime =
LocalDateTime.of(year, month, day, hours, minutes, seconds, 0)
/**
* Formats this date by the specifed date format.
*/
inline fun Date.format(format: DateFormat = DEFAULT_DATE_FORMAT): String = format.format(this)
/**
* Converts this string to a date by the specified date format.
*/
inline fun String.toDate(format: DateFormat = DEFAULT_DATE_FORMAT): Date = format.parse(this)
/**
* Parses this string to a duration.
*/
inline fun String.toDuration(): Duration = Duration.parse(this)
/**
* Converts this duration to a date.
*/
inline fun Duration.toDate(): Date = (inWholeMilliseconds + currentMillis).toDate()
/**
* Formats this duration. This is specified to:
* `duration.toDate().format()`
*/
inline fun Duration.format(format: DateFormat = DEFAULT_DATE_FORMAT): String = toDate().format(format)
/**
* Creates a duration of nanoseconds by the specified [value].
*/
inline fun nanoseconds(value: Long) = Duration.nanoseconds(value)
/**
* Creates a duration of microseconds by the specified [value].
*/
inline fun microseconds(value: Long) = Duration.microseconds(value)
/**
* Creates a duration of milliseconds by the specified [value].
*/
inline fun milliseconds(value: Long) = Duration.milliseconds(value)
/**
* Creates a duration of seconds by the specified [value].
*/
inline fun seconds(value: Long) = Duration.seconds(value)
/**
* Creates a duration of minutes by the specified [value].
*/
inline fun minutes(value: Long) = Duration.minutes(value)
/**
* Creates a duration of hours by the specified [value].
*/
inline fun hours(value: Long) = Duration.hours(value)
/**
* Creates a duration of days by the specified [value].
*/
inline fun days(value: Long) = Duration.days(value)
/**
* Creates a duration of nanoseconds by the specified [value].
*/
inline fun nanoseconds(value: Int) = Duration.nanoseconds(value)
/**
* Creates a duration of microseconds by the specified [value].
*/
inline fun microseconds(value: Int) = Duration.microseconds(value)
/**
* Creates a duration of milliseconds by the specified [value].
*/
inline fun milliseconds(value: Int) = Duration.milliseconds(value)
/**
* Creates a duration of seconds by the specified [value].
*/
inline fun seconds(value: Int) = Duration.seconds(value)
/**
* Creates a duration of minutes by the specified [value].
*/
inline fun minutes(value: Int) = Duration.minutes(value)
/**
* Creates a duration of hours by the specified [value].
*/
inline fun hours(value: Int) = Duration.hours(value)
/**
* Creates a duration of days by the specified [value].
*/
inline fun days(value: Int) = Duration.days(value)
/**
* Creates a duration of nanoseconds by the specified [value].
*/
inline fun nanoseconds(value: Double) = Duration.nanoseconds(value)
/**
* Creates a duration of microseconds by the specified [value].
*/
inline fun microseconds(value: Double) = Duration.microseconds(value)
/**
* Creates a duration of milliseconds by the specified [value].
*/
inline fun milliseconds(value: Double) = Duration.milliseconds(value)
/**
* Creates a duration of seconds by the specified [value].
*/
inline fun seconds(value: Double) = Duration.seconds(value)
/**
* Creates a duration of minutes by the specified [value].
*/
inline fun minutes(value: Double) = Duration.minutes(value)
/**
* Creates a duration of hours by the specified [value].
*/
inline fun hours(value: Double) = Duration.hours(value)
/**
* Creates a duration of days by the specified [value].
*/
inline fun days(value: Double) = Duration.days(value)
/**
* Adds the given [duration] to this date time.
*/
operator fun DateTime.plus(duration: Duration): DateTime {
return this + duration.inWholeMilliseconds.milliseconds
}
/**
* Creates a date progression by steping with the specified [step].
*/
infix fun ClosedRange.step(step: TimeSpan): DateProgression {
return DateProgression(this.start, this.endInclusive, step)
}
/**
* Creates a date progression by steping with the specified [step].
*/
infix fun ClosedRange.step(step: MonthSpan): DateMonthProgression {
return DateMonthProgression(this.start, this.endInclusive, step)
}
/**
* A date time progression range.
*/
class DateProgression(
override val start: DateTime,
override val endInclusive: DateTime,
val step: TimeSpan,
) : ClosedRange, Iterable {
override fun iterator(): Iterator {
return DateProgressionIterator(start, endInclusive, step)
}
}
/**
* A date time progression range with month span.
*/
class DateMonthProgression(
override val start: DateTime,
override val endInclusive: DateTime,
val step: MonthSpan,
) : ClosedRange, Iterable {
override fun iterator(): Iterator {
return DateProgressionMonthIterator(start, endInclusive, step)
}
}
class DateProgressionIterator(
var first: DateTime,
var last: DateTime,
val step: TimeSpan,
) : Iterator {
val finalElement = last
var hasNext = first <= last
var next = if (hasNext()) first else finalElement
override fun hasNext(): Boolean = hasNext
override fun next(): DateTime {
val value = next
val to = value + step
if (to >= finalElement) {
if (!hasNext) throw kotlin.NoSuchElementException()
hasNext = false
} else {
next = to
}
return value
}
}
class DateProgressionMonthIterator(
var first: DateTime,
var last: DateTime,
val step: MonthSpan,
) : Iterator {
val finalElement = last
var hasNext = first <= last
var next = if (hasNext()) first else finalElement
override fun hasNext(): Boolean = hasNext
override fun next(): DateTime {
val value = next
val to = value + step
if (to >= finalElement) {
if (!hasNext) throw kotlin.NoSuchElementException()
hasNext = false
} else {
next = to
}
return value
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy