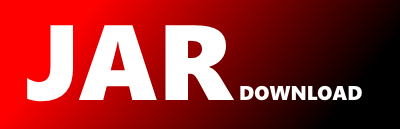
walkmc.extensions.numbers.Maths.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of walk-server Show documentation
Show all versions of walk-server Show documentation
A spigot fork to kotlin structure and news.
@file:Suppress("NOTHING_TO_INLINE")
package walkmc.extensions.numbers
import walkmc.extensions.collections.*
import kotlin.math.*
import kotlin.random.*
import java.math.*
/**
* Generates a random int value by the random instance of [Random].
*/
fun randomInt(): Int = Random.nextInt()
/**
* Generates a random int value with [max] by the random instance of [Random].
*/
fun randomInt(max: Int): Int = Random.nextInt(max)
/**
* Generates a random int value with [range] by the random instance of [Random].
*/
fun randomInt(range: IntRange): Int = Random.nextInt(range)
/**
* Generates a random int value and then converts into a byte by the random instance of [Random].
*/
fun randomByte(): Byte = Random.nextInt().toByte()
/**
* Generates a random int value with [max] and then converts into a byte by the random instance of [Random].
*/
fun randomByte(max: Byte): Byte = randomInt(max.toInt()).toByte()
/**
* Generates a random int value with [range] and then converts into a byte by the random instance of [Random].
*/
fun randomByte(range: IntRange): Byte = randomInt(range).toByte()
/**
* Generates a random int value and then converts into a short by the random instance of [Random].
*/
fun randomShort(): Short = Random.nextInt().toShort()
/**
* Generates a random int value with [max] and then converts into a short by the random instance of [Random].
*/
fun randomShort(max: Short): Short = randomInt(max.toInt()).toShort()
/**
* Generates a random int value with [range] and then converts into a short by the random instance of [Random].
*/
fun randomShort(range: IntRange): Short = randomInt(range).toShort()
/**
* Generates a random long value by the random instance of [Random].
*/
fun randomLong(): Long = Random.nextLong()
/**
* Generates a random long value with [max] by the random instance of [Random].
*/
fun randomLong(max: Long): Long = Random.nextLong(max)
/**
* Generates a random long value with [range] by the random instance of [Random].
*/
fun randomLong(range: LongRange): Long = Random.nextLong(range)
/**
* Generates a random boolean value by the random instance of [Random].
*/
fun randomBoolean(): Boolean = Random.nextBoolean()
/**
* Generates a random float value by the random instance of [Random].
*/
fun randomFloat(): Float = Random.nextFloat()
/**
* Generates a random float value by the random instance of [Random].
*/
fun randomFloat(max: Float): Float = Random.nextDouble(max.toDouble()).toFloat()
/**
* Generates a random float value by the random instance of [Random].
*/
fun randomFloat(range: FloatRange): Float =
Random.nextDouble(range.start.toDouble(), range.endInclusive.toDouble()).toFloat()
/**
* Generates a random double value by the random instance of [Random].
*/
fun randomDouble(): Double = Random.nextDouble()
/**
* Generates a random double value by the random instance of [Random].
*/
fun randomDouble(max: Double): Double = randomDouble(0.0..max)
/**
* Generates a random double value by the random instance of [Random].
*/
fun randomDouble(range: DoubleRange): Double = Random.nextDouble(range.start, range.endInclusive)
/**
* Generates a random chance by the specified percentage. For example:
* ```
* if (chance(35.0)) // has 35% of chance to return true
* println("35% of chance")
* ```
*/
fun chance(percentage: Double): Boolean = randomDouble() * 100 <= percentage
/**
* Returns a random number, specified by the [Math.random]
*/
inline fun random(): Double = Math.random()
/**
* Returns a random number between two adjacents min and max.
*/
fun randomBetween(min: Int, max: Int): Int = (min + (max - min) * random()).toInt()
/**
* Returns a random number between two adjacents min and max.
*/
fun randomBetween(min: Float, max: Float): Float = (min + (max - min) * random()).toFloat()
/**
* Returns a random number between two adjacents min and max.
*/
fun randomBetween(min: Double, max: Double): Double = min + (max - min) * random()
/**
* Returns the percentage needed to the [min] needs to get in [max].
*/
fun percentage(min: Number, max: Number) = min.toDouble() * 100 / max.toDouble()
/**
* Returns a number between two adjacents min and max.
* This is equals to
* ```
* max(min, min(number, max))
* ```
*/
inline fun between(min: Int, number: Int, max: Int): Int = max(min, min(number, max))
/**
* Returns a number between two adjacents min and max.
* This is equals to
* ```
* max(min, min(number, max))
* ```
*/
inline fun between(min: Float, number: Float, max: Float): Float = max(min, min(number, max))
/**
* Returns a number between two adjacents min and max.
* This is equals to
* ```
* max(min, min(number, max))
* ```
*/
inline fun between(min: Double, number: Double, max: Double): Double = max(min, min(number, max))
/**
* Plus a percent of this actual number.
*/
fun Number.plusPercent(percent: Double): Double = toDouble() + toDouble() / 100 * percent
/**
* Subtract a percent of this actual number.
*/
fun Number.minusPercent(percent: Double): Double = toDouble() - toDouble() / 100 * percent
/**
* Multiplies a percent of this actual number.
*/
fun Number.timesPercent(percent: Double): Double = toDouble() * toDouble() / 100 * percent
/**
* Divides a percent of this actual number.
*/
fun Number.divPercent(percent: Double): Double = toDouble() / toDouble() / 100 * percent
/**
* Rounds this double to a max decimal places.
*/
fun Double.decimals(decimals: Int): Double =
BigDecimal(this).setScale(decimals, RoundingMode.HALF_UP).toDouble()
/**
* Rounds this float to a max decimal places.
*/
fun Float.decimals(decimals: Int): Float =
BigDecimal(toDouble()).setScale(decimals, RoundingMode.HALF_UP).toFloat()
/**
* Returns a positive representation of this byte value if the value is negative.
*/
inline val Byte.positive get() = +this
/**
* Returns a negative representation of this byte value if the value is positive.
*/
inline val Byte.negative get() = -this
/**
* Returns a positive representation of this short value if the value is negative.
*/
inline val Short.positive get() = +this
/**
* Returns a negative representation of this short value if the value is positive.
*/
inline val Short.negative get() = -this
/**
* Returns a positive representation of this int value if the value is negative.
*/
inline val Int.positive get() = +this
/**
* Returns a negative representation of this int value if the value is positive.
*/
inline val Int.negative get() = -this
/**
* Returns a positive representation of this long value if the value is negative.
*/
inline val Long.positive get() = +this
/**
* Returns a negative representation of this long value if the value is positive.
*/
inline val Long.negative get() = -this
/**
* Returns a positive representation of this float value if the value is negative.
*/
inline val Float.positive get() = +this
/**
* Returns a negative representation of this float value if the value is positive.
*/
inline val Float.negative get() = -this
/**
* Returns a positive representation of this double value if the value is negative.
*/
inline val Double.positive get() = +this
/**
* Returns a negative representation of this double value if the value is positive.
*/
inline val Double.negative get() = -this
© 2015 - 2025 Weber Informatics LLC | Privacy Policy