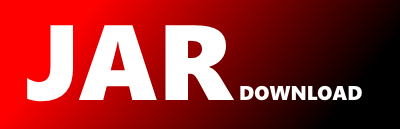
walkmc.library.LibraryManager.kt Maven / Gradle / Ivy
package walkmc.library
import walkmc.*
import walkmc.cache.*
import walkmc.extensions.*
import walkmc.serializer.common.*
import java.io.*
import java.lang.reflect.*
import java.net.*
/**
* A registry used to store and register libraries.
*/
object LibraryManager : Cache() {
const val MAVEN_CENTRAL = "https://repo.maven.apache.org/maven2/"
/**
* Gets the default folder of the libaries.
*/
val folder = newFolder("libraries")
/**
* Loads and register all libraries from the default library folder.
*/
fun loadAll() = folder
.files { extension == "jar" }
.filter { it.nameWithoutExtension !in this }
.forEach {
put(it.nameWithoutExtension, it)
addURLMethod.invoke(mainClassLoader, it.toURI().toURL())
}
/**
* Adds and register a new library by the specified [file].
*/
fun register(file: File) {
if (file.nameWithoutExtension in this)
return
file.copyTo(folder)
put(file.nameWithoutExtension, file)
addURLMethod.invoke(mainClassLoader, file.toURI().toURL())
}
/**
* Downloads a jar file from the specified [url] and implements then inside of the library folder.
* This alsos register the downloaded file in this registry.
*/
fun download(url: String): File {
val file = Downloader.download(URL(url), folder, true)
put(file.nameWithoutExtension, file)
addURLMethod.invoke(mainClassLoader, file.toURI().toURL())
return file
}
/**
* Downloads a jar file from the maven central and implements then inside of the library folder.
* This alsos register the downloaded file in this registry.
*/
fun downloadFromMaven(group: String, artifact: String, version: String): File {
val artifacts = artifact.replace('.', '/')
val groups = group.replace('.', '/')
val url = "$MAVEN_CENTRAL$groups/$artifacts/$version/$artifacts-$version.jar"
return download(url)
}
}
/**
* Gets the main class loader.
*/
internal val mainClassLoader by lazy {
ClassLoader.getSystemClassLoader() as URLClassLoader
}
/**
* Gets the java method used to add new URL libaries to the classpath.
*/
internal val addURLMethod: Method by lazy {
URLClassLoader::class.java.getDeclaredMethod("addURL", URL::class.java).apply {
isAccessible = true
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy