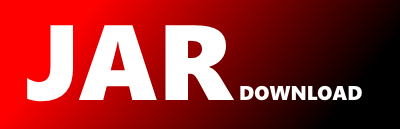
walkmc.time.Season.kt Maven / Gradle / Ivy
package walkmc.time
import com.soywiz.klock.*
/**
* A season enum that contains some utilities to get the current season of a [DateTime].
*/
enum class Season(val display: String) {
AUTUMN("Outono"),
WINTER("Inverno"),
SPRING("Primavera"),
SUMMER("Verão");
companion object {
val SEASONS = values()
/**
* Returns the season by the specified index.
*/
fun fromIndex(index: Int): Season {
return SEASONS[index]
}
/**
* Returns a season by the specified date.
*/
fun from(date: DateTime): Season {
return when {
date.isAutumn -> AUTUMN
date.isWinter -> WINTER
date.isSpring -> SPRING
date.isSummer -> SUMMER
else -> SUMMER
}
}
/**
* Returns the current season.
*/
fun fromNow(): Season {
return from(DateTime.now())
}
}
}
/**
* Returns the current season of this date time.
*/
fun DateTime.season(): Season {
return Season.from(this)
}
/**
* Verify if this date time is in autumn.
*/
val DateTime.isAutumn: Boolean get() {
return when (month) {
Month.April, Month.May -> true
Month.March -> dayOfMonth >= 21
Month.June -> dayOfMonth <= 21
else -> false
}
}
/**
* Verify if this date time is in winter.
*/
val DateTime.isWinter: Boolean get() {
return when (month) {
Month.July, Month.August -> true
Month.June -> dayOfMonth > 21
Month.September -> dayOfMonth <= 23
else -> false
}
}
/**
* Verify if this date time is in spring.
*/
val DateTime.isSpring: Boolean get() {
return when (month) {
Month.October, Month.November -> true
Month.September -> dayOfMonth > 23
Month.December -> dayOfMonth <= 21
else -> false
}
}
/**
* Verify if this date time is in summer.
*/
val DateTime.isSummer: Boolean get() {
return when (month) {
Month.January, Month.February -> true
Month.December -> dayOfMonth > 21
Month.March -> dayOfMonth <= 20
else -> false
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy