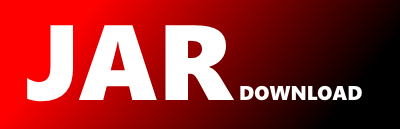
pickleib.utilities.element.acquisition.ElementAcquisition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Pickleib Show documentation
Show all versions of Pickleib Show documentation
Pickleib library for supporting web application automation with Selenium
The newest version!
package pickleib.utilities.element.acquisition;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import context.ContextStore;
import org.openqa.selenium.By;
import org.openqa.selenium.NoSuchElementException;
import org.openqa.selenium.WebDriverException;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.openqa.selenium.support.pagefactory.ByAll;
import pickleib.enums.PrimarySelectorType;
import pickleib.enums.SelectorType;
import pickleib.exceptions.PickleibException;
import pickleib.utilities.interfaces.repository.PageRepository;
import collections.Bundle;
import collections.Pair;
import utils.Printer;
import utils.StringUtilities;
import utils.arrays.lambda.Collectors;
import utils.reflection.ReflectionUtilities;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.util.*;
import static utils.StringUtilities.*;
import static utils.reflection.ReflectionUtilities.getFieldValue;
import static utils.reflection.ReflectionUtilities.getFields;
import static utils.StringUtilities.Color.*;
@SuppressWarnings("unused")
public class ElementAcquisition {
static long elementTimeout = Long.parseLong(ContextStore.get("element-timeout", "15000"));
static Printer log = new Printer(ElementAcquisition.class);
/**
* Acquire listed component by the text of its given child element
*
* @param items list of components
* @param attributeName component element attribute name
* @param attributeValue attribute value
* @param elementFieldName component elements field name
* @return returns the matching component
* @param component type
*/
public static Component acquireComponentByElementAttributeAmongst(
List items,
String attributeName,
String attributeValue,
String elementFieldName
){
log.info("Acquiring component by attribute " + highlighted(BLUE, attributeName + " -> " + attributeValue));
boolean timeout = false;
long initialTime = System.currentTimeMillis();
while (!timeout){
for (Component component : items) {
Map componentFields = getFields(component);
WebElement element = (WebElement) componentFields.get(elementFieldName);
String attribute = element.getAttribute(attributeName);
if (attribute.equals(attributeValue)) return component;
}
if (System.currentTimeMillis() - initialTime > elementTimeout) timeout = true;
}
throw new NoSuchElementException("No component with " + attributeName + " : " + attributeValue + " could be found!");
}
/**
* Acquire a listed element by its attribute
*
* @param items list that includes target element
* @param attributeName attribute name
* @param attributeValue attribute value
* @return returns the selected element
*/
public static WebElement acquireElementUsingAttributeAmongst(List items, String attributeName, String attributeValue){
log.info("Acquiring element called " + markup(BLUE, attributeValue) + " using its " + markup(BLUE, attributeName) + " attribute");
boolean condition = true;
boolean timeout = false;
long initialTime = System.currentTimeMillis();
WebDriverException caughtException = null;
int counter = 0;
while (!(System.currentTimeMillis() - initialTime > elementTimeout)){
try {
for (WebElement selection : items) {
String attribute = selection.getAttribute(attributeName);
if (attribute != null && (attribute.equalsIgnoreCase(attributeValue) || attribute.contains(attributeValue))) return selection;
}
}
catch (WebDriverException webDriverException){
if (counter != 0 && webDriverException.getClass().getName().equals(caughtException.getClass().getName()))
log.warning("Iterating... (" + webDriverException.getClass().getName() + ")");
caughtException = webDriverException;
counter++;
}
}
throw new NoSuchElementException("No element with the attributes '" + attributeName + " : " + attributeValue + "' could be found!");
}
/**
* Acquire listed element by its name
*
* @param items list that includes target element
* @param selectionName element name
* @return returns the selected element
*/
public static WebElement acquireNamedElementAmongst(List items, String selectionName){
boolean timeout = false;
long initialTime = System.currentTimeMillis();
WebDriverException caughtException = null;
int counter = 0;
do {
try {
for (WebElement selection : items) {
String text = selection.getText();
if (text.equalsIgnoreCase(selectionName) || text.contains(selectionName)) return selection;
}
}
catch (WebDriverException webDriverException){
if (counter == 0) {
log.warning("Iterating... (" + webDriverException.getClass().getName() + ")");
caughtException = webDriverException;
}
else if (!webDriverException.getClass().getName().equals(caughtException.getClass().getName())){
log.warning("Iterating... (" + webDriverException.getClass().getName() + ")");
caughtException = webDriverException;
}
counter++;
}
}
while (!(System.currentTimeMillis() - initialTime > elementTimeout));
throw new NoSuchElementException("No element with text/name '" + selectionName + "' could be found!");
}
/**
* Acquire a component amongst a list of components by its name
*
* @param items list of components
* @param selectionName component name
* @return returns the selected component
*/
public static Component acquireNamedComponentAmongst(
List items,
String selectionName
){
log.info("Acquiring component called " + highlighted(BLUE, selectionName));
boolean timeout = false;
long initialTime = System.currentTimeMillis();
WebDriverException caughtException = null;
int counter = 0;
while (!(System.currentTimeMillis() - initialTime > elementTimeout)){
try {
for (Component selection : items) {
String text = selection.getText();
if (text.equalsIgnoreCase(selectionName) || text.contains(selectionName)) return selection;
}
}
catch (WebDriverException webDriverException){
if (counter != 0 && webDriverException.getClass().getName().equals(caughtException.getClass().getName()))
log.warning("Iterating... (" + webDriverException.getClass().getName() + ")");
caughtException = webDriverException;
counter++;
}
}
throw new NoSuchElementException("No component with text/name '" + selectionName + "' could be found!");
}
public static class PageObjectModel {
Reflections reflections;
public PageObjectModel(Class pageRepository) {
reflections = new Reflections<>(pageRepository);
}
/**
*
* Acquire element {element name} from {page name}
*
* @param elementName target button name
* @param pageName specified page instance name
*/
public WebElement acquireElementFromPage(String elementName, String pageName){
log.info("Acquiring element " +
highlighted(BLUE, elementName) +
highlighted(GRAY," from the ") +
highlighted(BLUE, pageName)
);
pageName = firstLetterDeCapped(pageName);
elementName = contextCheck(elementName);
return reflections.getElementFromPage(elementName, pageName);
}
/**
*
* Acquire component element {element name} of {component field name} component on the {page name}
*
* @param elementName target button name
* @param componentFieldName specified component field name
* @param pageName specified page instance name
*/
public WebElement acquireElementFromComponent(String elementName, String componentFieldName, String pageName) {
log.info("Acquiring element " +
highlighted(BLUE, elementName) +
highlighted(GRAY," from the ") +
highlighted(BLUE, pageName)
);
pageName = firstLetterDeCapped(pageName);
componentFieldName = firstLetterDeCapped(componentFieldName);
elementName = contextCheck(elementName);
return reflections.getElementFromComponent(elementName, componentFieldName, pageName);
}
/**
*
* Acquire a listed element {element name} from {list name} list on the {page name}
*
* @param elementName target button name
* @param listName specified component list name
* @param pageName specified page instance name
*/
public WebElement acquireListedElementFromPage(
String elementName,
String listName,
String pageName
) {
log.info("Acquiring listed element named " +
highlighted(BLUE, elementName) +
highlighted(GRAY," selected from ") +
highlighted(BLUE, listName) +
highlighted(GRAY," on ") +
highlighted(BLUE, pageName)
);
pageName = firstLetterDeCapped(pageName);
listName = firstLetterDeCapped(listName);
elementName = contextCheck(elementName);
List elements = reflections.getElementsFromPage(
listName,
pageName
);
return acquireNamedElementAmongst(elements, elementName);
}
/**
*
* Acquire a listed component element {element name} of {component field name} from {component list name} list on the {page name}
*
* @param elementName target button name
* @param componentFieldName specified component field name
* @param listFieldName specified component list name
* @param pageName specified page instance name
*/
public WebElement acquireListedElementFromComponent(
String elementName,
String componentFieldName,
String listFieldName,
String pageName
) {
log.info("Acquiring listed element named " +
highlighted(BLUE, elementName) +
highlighted(GRAY," selected from ") +
highlighted(BLUE, listFieldName) +
highlighted(GRAY," of ") +
highlighted(BLUE, componentFieldName) +
highlighted(GRAY," component on the ") +
highlighted(BLUE, pageName)
);
componentFieldName = firstLetterDeCapped(componentFieldName);
pageName = firstLetterDeCapped(pageName);
listFieldName = firstLetterDeCapped(listFieldName);
elementName = contextCheck(elementName);
List elements = reflections.getElementsFromComponent(
listFieldName,
componentFieldName,
pageName
);
return acquireNamedElementAmongst(elements, elementName);
}
/**
*
* Select component named {component name} from {component list name} component list on the {page name} and acquire the {element name} element
*
* @param componentName specified component name
* @param componentListName specified component list name
* @param pageName specified page instance name
* @param elementName target button name
*/
public WebElement acquireListedComponentElement(
String elementName,
String componentName,
String componentListName,
String pageName
) {
log.info("Acquiring listed element named " +
highlighted(BLUE, elementName) +
highlighted(GRAY," selected from ") +
highlighted(BLUE, componentListName) +
highlighted(GRAY," on the ") +
highlighted(BLUE, componentName) +
highlighted(GRAY," component on the ") +
highlighted(BLUE, pageName)
);
pageName = firstLetterDeCapped(pageName);
componentListName = firstLetterDeCapped(componentListName);
elementName = contextCheck(elementName);
List componentList = reflections.getComponentsFromPage(componentListName, pageName);
Component component = acquireNamedComponentAmongst(componentList, componentName);
return reflections.getElementFromComponent(elementName, component);
}
/**
*
* Select exact component named {component name} from {component list name} component list on the {page name} and acquire the {element name} element
*
* @param elementFieldName specified element field name
* @param elementText specified element text
* @param componentListName specified component list name
* @param pageName specified page instance name
*/
public WebElement acquireExactNamedListedComponentElement(
String elementFieldName,
String elementText,
String componentListName,
String pageName
) {
log.info("Acquiring exact listed element named " +
highlighted(BLUE, elementFieldName) +
highlighted(GRAY," selected from ") +
highlighted(BLUE, componentListName) +
highlighted(GRAY," component list on the ") +
highlighted(BLUE, pageName)
);
Object component = acquireExactNamedListedComponent(elementFieldName, elementText, componentListName, pageName);
return reflections.getElementFromComponent(elementFieldName, component);
}
/**
*
* Acquire component {component name} from {component list name} component list on the {page name} and by selecting it using child element name
*
* @param elementText specified element text
* @param componentListName specified component list name
* @param pageName specified page instance name
* @param elementFieldName target element name
*/
public Component acquireExactNamedListedComponent(
String elementFieldName,
String elementText,
String componentListName,
String pageName
) {
log.info("Acquiring exact listed component by element named " +
highlighted(BLUE, elementFieldName) +
highlighted(GRAY," selected from ") +
highlighted(BLUE, componentListName) +
highlighted(GRAY," component list on the ") +
highlighted(BLUE, pageName)
);
pageName = firstLetterDeCapped(pageName);
componentListName = firstLetterDeCapped(componentListName);
elementFieldName = contextCheck(elementFieldName);
List components = reflections.getComponentsFromPage(componentListName, pageName);
return reflections.acquireExactNamedComponentAmongst(components, elementText, elementFieldName);
}
/**
*
* Select component named {component name} from {component list name} component list on the {page name} and acquire listed element {element name} of {element list name}
*
* @param componentName specified component name
* @param componentListName specified component list name
* @param pageName specified page instance name
* @param elementName target button name
* @param elementListName target element list name
*/
public WebElement acquireListedElementAmongstListedComponents(
String elementName,
String elementListName,
String componentName,
String componentListName,
String pageName
) {
log.info("Acquiring listed element named " +
highlighted(BLUE, elementName) +
highlighted(GRAY," selected from ") +
highlighted(BLUE, componentListName) +
highlighted(GRAY," component list on the ") +
highlighted(BLUE, pageName)
);
elementName = contextCheck(elementName);
componentName = contextCheck(componentName);
pageName = firstLetterDeCapped(pageName);
componentListName = firstLetterDeCapped(componentListName);
List components = reflections.getComponentsFromPage(componentListName, pageName);
Component component = acquireNamedComponentAmongst(components, componentName);
List elements = reflections.getElementsFromComponent(elementListName, component);
return acquireNamedElementAmongst(elements, elementName);
}
/**
*
* Acquire a listed attribute element that has {attribute value} value for its {attribute name} attribute from {list name} list on the {page name}
*
* @param attributeName target attribute name
* @param attributeValue expected attribute value
* @param listName target list name
* @param pageName specified page instance name
*/
public WebElement acquireListedElementByAttribute(
String attributeName,
String attributeValue,
String listName,
String pageName
) {
log.info("Acquiring element by " +
highlighted(BLUE, attributeName) +
highlighted(GRAY," attribute selected from ") +
highlighted(BLUE, listName) +
highlighted(GRAY, " list on the ") +
highlighted(BLUE, pageName)
);
attributeName = contextCheck(attributeName);
pageName = firstLetterDeCapped(pageName);
List elements = reflections.getElementsFromPage(
listName,
firstLetterDeCapped(pageName)
);
return acquireElementUsingAttributeAmongst(elements, attributeName, attributeValue);
}
/**
*
* Acquire listed attribute element of {component name} component that has {attribute value} value for its {attribute name} attribute from {list name} list on the {page name}
*
* @param componentName specified component name
* @param attributeValue expected attribute value
* @param attributeName target attribute name
* @param listName target list name
* @param pageName specified page instance name
*/
public WebElement acquireListedComponentElementByAttribute(
String componentName,
String attributeValue,
String attributeName,
String listName,
String pageName
) {
log.info("Acquiring element by " +
highlighted(BLUE, attributeName) +
highlighted(GRAY," attribute selected from ") +
highlighted(BLUE, listName) +
highlighted(GRAY, " list on the ") +
highlighted(BLUE, pageName)
);
attributeName = contextCheck(attributeName);
pageName = firstLetterDeCapped(pageName);
componentName = firstLetterDeCapped(componentName);
List elements = reflections.getElementsFromComponent(
listName,
componentName,
pageName
);
return acquireElementUsingAttributeAmongst(elements, attributeName, attributeValue);
}
/**
* Acquire form input on the {page name}
*
* @param pageName specified page instance name
* @param signForms table that has key as "Input" and value as "Input Element" (dataTable.asMaps())
*/
public List> acquireElementList(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy