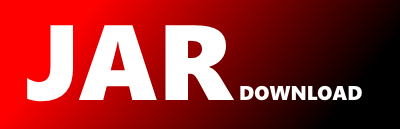
utils.SQLUtilities Maven / Gradle / Ivy
package utils;
import java.sql.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Deprecated(since = "1.2.2")
public class SQLUtilities {
private final Printer log = new Printer(SQLUtilities.class);
public Connection getConnection(String user, String password, String url, String database) {
log.new Info("Establishing database connection...");
if (database!=null) url += ";database=" + database;
try {
Connection connection = DriverManager.getConnection(url,user,password);
log.new Success("Connection established!");
return connection;
}
catch (SQLException e) {throw new RuntimeException(e);}
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy