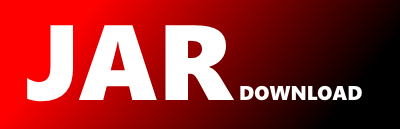
properties.UtilityPropertiesMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Utilities Show documentation
Show all versions of Utilities Show documentation
Java Utilities library containing general use utilities
package properties;
import java.util.*;
import static java.util.Objects.requireNonNull;
public class UtilityPropertiesMap extends AbstractMap {
final UtilityPropertiesMap parent;
final Map delegate;
UtilityPropertiesMap(UtilityPropertiesMap parent, Map delegate) {
this.delegate = requireNonNull(delegate);
this.parent = parent;
}
UtilityPropertiesMap(Map delegate) {
this(null, delegate);
}
@Override
public Set> entrySet() {
return delegate.entrySet();
}
/**
* Creates a UtilityPropertiesMap from a Properties object.
*
* @param p The Properties object to create the map from.
* @return A UtilityPropertiesMap created from the Properties object.
*/
static UtilityPropertiesMap create(Properties p) {
Map copy = new HashMap<>();
p.stringPropertyNames().forEach(s -> copy.put(s, p.getProperty(s)));
return new UtilityPropertiesMap(copy);
}
@Override
public String get(Object key) {
String exactMatch = super.get(key);
if (exactMatch != null) {
return exactMatch;
}
if (!(key instanceof String keyString)) {
return null;
}
String formattedKey = formatKey(keyString);
String formattedMatch = super.get(formattedKey);
if (formattedMatch != null) {
return formattedMatch;
}
if (parent == null) {
return null;
}
return parent.get(key);
}
/**
* Formats a key to handle variations in case and characters.
*
* @param key The key to format.
* @return The formatted key.
*/
String formatKey(String key) {
return key
.replace(".", "_")
.replace("-", "_")
.toLowerCase(Locale.ENGLISH);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy