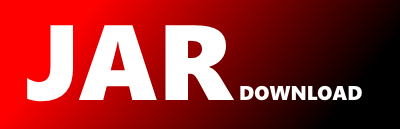
io.github.vipcxj.jasync.spec.JAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jasync-spec Show documentation
Show all versions of jasync-spec Show documentation
JAsync implements Async-Await fashion for Java just like es and c#.
This library provide the spec of all public api.
package io.github.vipcxj.jasync.spec;
import io.github.vipcxj.jasync.spec.functional.*;
import io.github.vipcxj.jasync.spec.spi.PromiseProvider;
import io.github.vipcxj.jasync.spec.switchexpr.ICase;
import java.util.List;
public class JAsync {
private static final PromiseProvider provider = Utils.getProvider();
private static PromiseProvider assertProvider() {
if (provider == null) {
throw new IllegalStateException("No provider of PromiseProvider found!");
}
return provider;
}
public static JPromise just() {
return assertProvider().just(null);
}
public static JPromise just(T value) {
return assertProvider().just(value);
}
public static JPromise defer(PromiseSupplier block) {
return assertProvider().defer(block);
}
public static JPromise deferVoid(VoidPromiseSupplier block) {
return defer(block);
}
public static JPromise deferVoid(VoidPromiseSupplier block, String label) {
return defer(block).doCatch(BreakException.class, e -> e.matchLabel(label, true) ? null : JAsync.error(e));
}
public static JPromise error(Throwable t) {
return assertProvider().error(t);
}
public static JPromise doIf(boolean test, VoidPromiseSupplier thenDo, VoidPromiseSupplier elseDo) {
try {
if (test) {
return Utils.safeGetVoid(thenDo);
} else {
return Utils.safeGetVoid(elseDo);
}
} catch (Throwable e) {
return JAsync.error(e);
}
}
public static void doContinue(String label) {
throw new ContinueException(label);
}
public static void doBreak(String label) {
throw new BreakException(label);
}
public static JPromise doReturn(JPromise promise) {
if (promise != null) {
return promise.then(v -> error(new ReturnException(v)));
} else {
return error(new ReturnException(null));
}
}
public static JPromise doSwitch(C value, List extends ICase> cases, String label) {
return just().doSwitch(value, cases, label);
}
public static JPromise doSwitch(C value, List extends ICase> cases) {
return just().doSwitch(value, cases);
}
public static JPromise doWhile(BooleanSupplier predicate, VoidPromiseSupplier block, String label) {
return just().doWhileVoid(predicate, block, label);
}
public static JPromise doWhile(BooleanSupplier predicate, VoidPromiseSupplier block) {
return just().doWhileVoid(predicate, block);
}
public static JPromise doWhile(PromiseSupplier predicate, VoidPromiseSupplier block, String label) {
return just().doWhileVoid(predicate, block, label);
}
public static JPromise doWhile(PromiseSupplier predicate, VoidPromiseSupplier block) {
return just().doWhileVoid(predicate, block);
}
public static JPromise doDoWhile(BooleanSupplier predicate, VoidPromiseSupplier block, String label) {
return just().doDoWhileVoid(predicate, block, label);
}
public static JPromise doDoWhile(BooleanSupplier predicate, VoidPromiseSupplier block) {
return just().doDoWhileVoid(predicate, block);
}
public static JPromise doDoWhile(PromiseSupplier predicate, VoidPromiseSupplier block, String label) {
return just().doDoWhileVoid(predicate, block, label);
}
public static JPromise doDoWhile(PromiseSupplier predicate, VoidPromiseSupplier block) {
return just().doDoWhileVoid(predicate, block);
}
public static JPromise doForEachObject(Object iterableOrArray, VoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray.getClass().isArray() && !iterableOrArray.getClass().getComponentType().isPrimitive()) {
//noinspection unchecked
return just().doForEachObjectArray((E[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block, label);
} else {
throw new IllegalArgumentException("The iterable must be object array or iterable, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachObject(Object iterableOrArray, VoidPromiseFunction block) {
return doForEachObject(iterableOrArray, block, null);
}
public static JPromise doForEachByte(Object iterableOrArray, ByteVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof byte[]) {
return just().doForEachByteArray((byte[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be byte array or iterable of Byte, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachByte(Object iterableOrArray, ByteVoidPromiseFunction block) {
return doForEachByte(iterableOrArray, block, null);
}
public static JPromise doForEachChar(Object iterableOrArray, CharVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof char[]) {
return just().doForEachCharArray((char[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be char array or iterable of Character, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachChar(Object iterableOrArray, CharVoidPromiseFunction block) {
return doForEachChar(iterableOrArray, block, null);
}
public static JPromise doForEachBoolean(Object iterableOrArray, BooleanVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof boolean[]) {
return just().doForEachBooleanArray((boolean[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be boolean array or iterable of Boolean, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachBoolean(Object iterableOrArray, BooleanVoidPromiseFunction block) {
return doForEachBoolean(iterableOrArray, block, null);
}
public static JPromise doForEachShort(Object iterableOrArray, ShortVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof short[]) {
return just().doForEachShortArray((short[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be short array or iterable of Short, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachShort(Object iterableOrArray, ShortVoidPromiseFunction block) {
return doForEachShort(iterableOrArray, block, null);
}
public static JPromise doForEachInt(Object iterableOrArray, IntVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof int[]) {
return just().doForEachIntArray((int[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be int array or iterable of Integer, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachInt(Object iterableOrArray, IntVoidPromiseFunction block) {
return doForEachInt(iterableOrArray, block, null);
}
public static JPromise doForEachLong(Object iterableOrArray, LongVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof long[]) {
return just().doForEachLongArray((long[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be long array or iterable of Long, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachLong(Object iterableOrArray, LongVoidPromiseFunction block) {
return doForEachLong(iterableOrArray, block, null);
}
public static JPromise doForEachFloat(Object iterableOrArray, FloatVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof float[]) {
return just().doForEachFloatArray((float[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be float array or iterable of Float, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachFloat(Object iterableOrArray, FloatVoidPromiseFunction block) {
return doForEachFloat(iterableOrArray, block, null);
}
public static JPromise doForEachDouble(Object iterableOrArray, DoubleVoidPromiseFunction block, String label) {
if (iterableOrArray == null) {
return just();
} else if (iterableOrArray instanceof double[]) {
return just().doForEachDoubleArray((double[]) iterableOrArray, block, label);
} else if (iterableOrArray instanceof Iterable) {
//noinspection unchecked
return just().doForEachIterable((Iterable) iterableOrArray, block::apply, label);
} else {
throw new IllegalArgumentException("The iterable must be double array or iterable of Double, but it is " + iterableOrArray.getClass().getName() + ".");
}
}
public static JPromise doForEachDouble(Object iterableOrArray, DoubleVoidPromiseFunction block) {
return doForEachDouble(iterableOrArray, block, null);
}
private static JPromise doForBody(VoidPromiseSupplier step, VoidPromiseSupplier body) throws Throwable {
return Utils.safeGetVoid(body).doFinally(() -> {
Utils.safeGetVoid(step);
return null;
});
}
public static JPromise doFor(VoidPromiseSupplier init, BooleanSupplier cond, VoidPromiseSupplier step, VoidPromiseSupplier body, String label) {
if (cond == null) {
cond = () -> true;
}
try {
return Utils.safeGetVoid(init).doWhileVoid(cond, () -> doForBody(step, body), label);
} catch (Throwable t) {
return JAsync.error(t);
}
}
public static JPromise doFor(VoidPromiseSupplier init, PromiseSupplier cond, VoidPromiseSupplier step, VoidPromiseSupplier body, String label) {
if (cond == null) {
cond = () -> JAsync.just(true);
}
try {
return Utils.safeGetVoid(init).doWhileVoid(cond, () -> doForBody(step, body), label);
} catch (Throwable t) {
return JAsync.error(t);
}
}
public static boolean mustRethrowException(Throwable t, List extends Class extends Throwable>> exceptionsType) {
if (t instanceof ReturnException) {
return exceptionsType.stream().noneMatch(ReturnException.class::equals);
}
if (t instanceof BreakException) {
return exceptionsType.stream().noneMatch(BreakException.class::equals);
}
if (t instanceof ContinueException) {
return exceptionsType.stream().noneMatch(ContinueException.class::equals);
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy