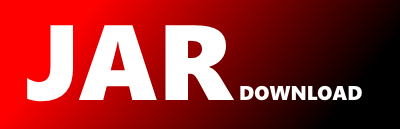
io.github.vipcxj.jasync.spec.JPromise Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jasync-spec Show documentation
Show all versions of jasync-spec Show documentation
JAsync implements Async-Await fashion for Java just like es and c#.
This library provide the spec of all public api.
package io.github.vipcxj.jasync.spec;
import io.github.vipcxj.jasync.spec.catcher.Catcher;
import io.github.vipcxj.jasync.spec.functional.*;
import io.github.vipcxj.jasync.spec.switchexpr.ICase;
import java.time.Duration;
import java.util.Collections;
import java.util.List;
public interface JPromise {
default T await() {
throw new UnsupportedOperationException("The method \"JPromise#await\" should be invoked in an async method. " +
"An async method is a method annotated with @JAsync and returning the JPromise object. " +
"The method should not be a lambda method or in an anonymous class");
}
JPromise then(PromiseFunction resolver);
default JPromise then(PromiseSupplier resolver) {
return this.then(ignored -> resolver.get());
}
default JPromise thenVoid(VoidPromiseFunction resolver) {
return this.then(resolver);
}
default JPromise thenVoid(VoidPromiseSupplier resolver) {
return this.then(resolver);
}
default JPromise thenVoid() {
return this.then(() -> null);
}
JPromise doCatch(List> exceptionsType, PromiseFunction reject);
default JPromise doCatch(List> exceptionsType, PromiseSupplier reject) {
return this.doCatch(exceptionsType, ignored -> {
return reject.get();
});
}
default JPromise doCatch(List> exceptionsType, ThrowableConsumer reject) {
return this.doCatch(exceptionsType, t -> {
reject.accept(t);
return null;
});
}
default JPromise doCatch(Class exceptionType, PromiseFunction reject) {
return doCatch(Collections.singletonList(exceptionType), t -> {
//noinspection unchecked
return reject.apply((E) t);
});
}
default JPromise doCatch(Class exceptionType, PromiseSupplier reject) {
return this.doCatch(exceptionType, ignored -> {
return reject.get();
});
}
default JPromise doCatch(Class exceptionType, ThrowableConsumer reject) {
return this.doCatch(exceptionType, t -> {
reject.accept(t);
return null;
});
}
default JPromise doCatch(PromiseFunction reject) {
return this.doCatch(Collections.singletonList(Throwable.class), reject);
}
default JPromise doCatch(PromiseSupplier reject) {
return this.doCatch(Collections.singletonList(Throwable.class), ignored -> {
return reject.get();
});
}
default JPromise doCatch(ThrowableConsumer reject) {
return this.doCatch(Collections.singletonList(Throwable.class), t -> {
reject.accept(t);
return null;
});
}
JPromise doCatch(List> catchers);
JPromise doFinally(VoidPromiseSupplier block);
JPromise doWhile(BooleanSupplier predicate, PromiseFunction block, String label);
JPromise doWhileVoid(BooleanSupplier predicate, VoidPromiseSupplier block, String label);
JPromise doWhile(PromiseSupplier predicate, PromiseFunction block, String label);
JPromise doWhileVoid(PromiseSupplier predicate, VoidPromiseSupplier block, String label);
default JPromise doDoWhile(BooleanSupplier predicate, PromiseFunction block, String label) {
return this.then(v -> Utils.safeApply(block, v).doCatch(ContinueException.class, e -> {
if (e.matchLabel(label)) {
return null;
}
return JAsync.error(e);
}).doWhile(predicate, block, label));
}
default JPromise doDoWhileVoid(BooleanSupplier predicate, VoidPromiseSupplier block, String label) {
return this.thenVoid(() -> Utils.safeGetVoid(block).doCatch(ContinueException.class, e -> {
if (e.matchLabel(label)) {
return null;
}
return JAsync.error(e);
}).doWhileVoid(predicate, block, label));
}
default JPromise doDoWhile(PromiseSupplier predicate, PromiseFunction block, String label) {
return this.then(v -> Utils.safeApply(block, v).doCatch(ContinueException.class, e -> {
if (e.matchLabel(label)) {
return null;
}
return JAsync.error(e);
}).doWhile(predicate, block, label));
}
default JPromise doDoWhileVoid(PromiseSupplier predicate, VoidPromiseSupplier block, String label) {
return this.thenVoid(() -> Utils.safeGetVoid(block).doCatch(ContinueException.class, e -> {
if (e.matchLabel(label)) {
return null;
}
return JAsync.error(e);
}).doWhileVoid(predicate, block, label));
}
default JPromise doWhile(BooleanSupplier predicate, PromiseFunction block) {
return doWhile(predicate, block, null);
}
default JPromise doWhileVoid(BooleanSupplier predicate, VoidPromiseSupplier block) {
return doWhileVoid(predicate, block, null);
}
default JPromise doWhile(PromiseSupplier predicate, PromiseFunction block) {
return doWhile(predicate, block, null);
}
default JPromise doWhileVoid(PromiseSupplier predicate, VoidPromiseSupplier block) {
return doWhileVoid(predicate, block, null);
}
default JPromise doDoWhile(BooleanSupplier predicate, PromiseFunction block) {
return doDoWhile(predicate, block, null);
}
default JPromise doDoWhileVoid(BooleanSupplier predicate, VoidPromiseSupplier block) {
return doDoWhileVoid(predicate, block, null);
}
default JPromise doDoWhile(PromiseSupplier predicate, PromiseFunction block) {
return doDoWhile(predicate, block, null);
}
default JPromise doDoWhileVoid(PromiseSupplier predicate, VoidPromiseSupplier block) {
return doDoWhileVoid(predicate, block, null);
}
JPromise doForEachIterable(Iterable iterable, VoidPromiseFunction block, String label);
JPromise doForEachObjectArray(E[] array, VoidPromiseFunction block, String label);
JPromise doForEachByteArray(byte[] array, ByteVoidPromiseFunction block, String label);
JPromise doForEachCharArray(char[] array, CharVoidPromiseFunction block, String label);
JPromise doForEachBooleanArray(boolean[] array, BooleanVoidPromiseFunction block, String label);
JPromise doForEachShortArray(short[] array, ShortVoidPromiseFunction block, String label);
JPromise doForEachIntArray(int[] array, IntVoidPromiseFunction block, String label);
JPromise doForEachLongArray(long[] array, LongVoidPromiseFunction block, String label);
JPromise doForEachFloatArray(float[] array, FloatVoidPromiseFunction block, String label);
JPromise doForEachDoubleArray(double[] array, DoubleVoidPromiseFunction block, String label);
default JPromise doForEachIterable(Iterable iterable, VoidPromiseFunction block) {
return doForEachIterable(iterable, block, null);
}
default JPromise doForEachObjectArray(E[] array, VoidPromiseFunction block) {
return doForEachObjectArray(array, block, null);
}
default JPromise doForEachByteArray(byte[] array, ByteVoidPromiseFunction block) {
return doForEachByteArray(array, block, null);
}
default JPromise doForEachCharArray(char[] array, CharVoidPromiseFunction block) {
return doForEachCharArray(array, block, null);
}
default JPromise doForEachBooleanArray(boolean[] array, BooleanVoidPromiseFunction block) {
return doForEachBooleanArray(array, block, null);
}
default JPromise doForEachShortArray(short[] array, ShortVoidPromiseFunction block) {
return doForEachShortArray(array, block, null);
}
default JPromise doForEachIntArray(int[] array, IntVoidPromiseFunction block) {
return doForEachIntArray(array, block, null);
}
default JPromise doForEachLongArray(long[] array, LongVoidPromiseFunction block) {
return doForEachLongArray(array, block, null);
}
default JPromise doForEachFloatArray(float[] array, FloatVoidPromiseFunction block) {
return doForEachFloatArray(array, block, null);
}
default JPromise doForEachDoubleArray(double[] array, DoubleVoidPromiseFunction block) {
return doForEachDoubleArray(array, block, null);
}
JPromise doSwitch(C value, List extends ICase> cases, String label);
default JPromise doSwitch(C value, List extends ICase> cases) {
return doSwitch(value, cases, null);
}
JPromise catchReturn();
Handle async();
T block();
T block(Duration duration);
I unwrap(Class> type);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy