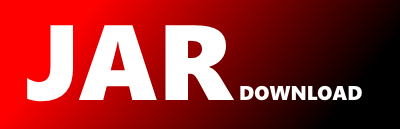
io.github.vipcxj.jasync.spec.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jasync-spec Show documentation
Show all versions of jasync-spec Show documentation
JAsync implements Async-Await fashion for Java just like es and c#.
This library provide the spec of all public api.
package io.github.vipcxj.jasync.spec;
import io.github.vipcxj.jasync.spec.functional.*;
import io.github.vipcxj.jasync.spec.spi.PromiseProvider;
import java.util.Iterator;
import java.util.ServiceLoader;
public class Utils {
public static PromiseProvider getProvider() {
ServiceLoader loader = ServiceLoader.load(PromiseProvider.class);
Iterator iterator = loader.iterator();
if (iterator.hasNext()) {
return iterator.next();
} else {
return null;
}
}
public static JPromise safeApply(PromiseFunction fuc, T v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(BooleanVoidPromiseFunction fuc, boolean v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(ByteVoidPromiseFunction fuc, byte v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(CharVoidPromiseFunction fuc, char v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(ShortVoidPromiseFunction fuc, short v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(IntVoidPromiseFunction fuc, int v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(LongVoidPromiseFunction fuc, long v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(FloatVoidPromiseFunction fuc, float v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeApply(DoubleVoidPromiseFunction fuc, double v) throws Throwable {
JPromise res = fuc != null ? fuc.apply(v) : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeGetVoid(VoidPromiseSupplier fuc) throws Throwable {
JPromise res = fuc != null ? fuc.get() : null;
return res != null ? res : JAsync.just();
}
public static JPromise safeGet(PromiseSupplier fuc) throws Throwable {
JPromise res = fuc != null ? fuc.get() : null;
return res != null ? res : JAsync.just();
}
public static boolean safeTest(BooleanSupplier fun) throws Throwable {
return fun != null && fun.getAsBoolean();
}
public static JPromise safeTest(PromiseSupplier fun) throws Throwable {
JPromise res = fun != null ? fun.get() : null;
return res != null ? res.then(test -> JAsync.just(Boolean.TRUE.equals(test))) : JAsync.just(false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy